用c语言写一份完整的学生信息管理系统 要求: (1)数据结构:创建学生信息结点类型以及学生信息结构体; (2)存储结构:选择单链表存储学生信息。 (3)实现功能(以函数方式实现):学生信息链表创建、学生信息输入、学生信息查询、学生信息修改、学生信息删除、学生信息输出(全部或部分输出)或保存到文件,学生相关排序。
时间: 2023-12-20 17:07:26 浏览: 66
好的,以下是一份使用C语言编写的学生信息管理系统,具体实现了您提出的要求:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct student_info {
int id;
char name[20];
int age;
struct student_info *next;
} StudentInfo;
StudentInfo *create_student_info(int id, char name[], int age) {
StudentInfo *new_student_info = (StudentInfo *)malloc(sizeof(StudentInfo));
new_student_info->id = id;
strcpy(new_student_info->name, name);
new_student_info->age = age;
new_student_info->next = NULL;
return new_student_info;
}
StudentInfo *add_student_info(StudentInfo *head, StudentInfo *new_student_info) {
if (head == NULL) {
head = new_student_info;
} else {
StudentInfo *current = head;
while (current->next != NULL) {
current = current->next;
}
current->next = new_student_info;
}
return head;
}
void print_student_info(StudentInfo *student_info) {
printf("%d\t%s\t%d\n", student_info->id, student_info->name, student_info->age);
}
void print_all_student_info(StudentInfo *head) {
StudentInfo *current = head;
printf("ID\tName\tAge\n");
while (current != NULL) {
print_student_info(current);
current = current->next;
}
}
StudentInfo *find_student_info(StudentInfo *head, int id) {
StudentInfo *current = head;
while (current != NULL) {
if (current->id == id) {
return current;
}
current = current->next;
}
return NULL;
}
StudentInfo *delete_student_info(StudentInfo *head, int id) {
StudentInfo *current = head;
if (current == NULL) {
printf("There is no student information to delete.\n");
return NULL;
}
if (current->id == id) {
head = current->next;
free(current);
printf("Delete student information with ID %d successfully.\n", id);
return head;
}
while (current->next != NULL) {
if (current->next->id == id) {
StudentInfo *temp = current->next;
current->next = temp->next;
free(temp);
printf("Delete student information with ID %d successfully.\n", id);
return head;
}
current = current->next;
}
printf("There is no student information with ID %d.\n", id);
return head;
}
void modify_student_info(StudentInfo *student_info) {
printf("Please enter the new information of the student:\n");
printf("ID: ");
scanf("%d", &student_info->id);
printf("Name: ");
scanf("%s", student_info->name);
printf("Age: ");
scanf("%d", &student_info->age);
}
int compare_by_id(StudentInfo *a, StudentInfo *b) {
return a->id - b->id;
}
int compare_by_name(StudentInfo *a, StudentInfo *b) {
return strcmp(a->name, b->name);
}
void sort_student_info(StudentInfo *head, int (*compare)(StudentInfo *a, StudentInfo *b)) {
int flag = 1;
while (flag) {
flag = 0;
StudentInfo *current = head;
while (current->next != NULL) {
if (compare(current, current->next) > 0) {
StudentInfo *temp = create_student_info(current->id, current->name, current->age);
modify_student_info(current);
modify_student_info(current->next);
temp->id = current->id;
strcpy(temp->name, current->name);
temp->age = current->age;
current->id = current->next->id;
strcpy(current->name, current->next->name);
current->age = current->next->age;
current->next->id = temp->id;
strcpy(current->next->name, temp->name);
current->next->age = temp->age;
flag = 1;
free(temp);
}
current = current->next;
}
}
}
void save_student_info_to_file(StudentInfo *head, char *filename) {
FILE *fp = fopen(filename, "w");
if (fp == NULL) {
printf("Failed to open file %s.\n", filename);
return;
}
StudentInfo *current = head;
while (current != NULL) {
fprintf(fp, "%d %s %d\n", current->id, current->name, current->age);
current = current->next;
}
fclose(fp);
printf("Save student information to file %s successfully.\n", filename);
}
StudentInfo *load_student_info_from_file(StudentInfo *head, char *filename) {
FILE *fp = fopen(filename, "r");
if (fp == NULL) {
printf("Failed to open file %s.\n", filename);
return head;
}
int id, age;
char name[20];
while (fscanf(fp, "%d %s %d", &id, name, &age) != EOF) {
StudentInfo *new_student_info = create_student_info(id, name, age);
head = add_student_info(head, new_student_info);
}
fclose(fp);
printf("Load student information from file %s successfully.\n", filename);
return head;
}
int main() {
StudentInfo *head = NULL;
while (1) {
printf("Please select the function you want to perform:\n");
printf("1. Create student information\n");
printf("2. Input student information\n");
printf("3. Query student information\n");
printf("4. Modify student information\n");
printf("5. Delete student information\n");
printf("6. Output student information\n");
printf("7. Save student information to file\n");
printf("8. Load student information from file\n");
printf("9. Sort student information by ID\n");
printf("10. Sort student information by name\n");
printf("0. Exit\n");
int choice;
scanf("%d", &choice);
switch (choice) {
case 0:
printf("Exit successfully.\n");
return 0;
case 1: {
int id, age;
char name[20];
printf("Please enter the student information:\n");
printf("ID: ");
scanf("%d", &id);
printf("Name: ");
scanf("%s", name);
printf("Age: ");
scanf("%d", &age);
StudentInfo *new_student_info = create_student_info(id, name, age);
head = add_student_info(head, new_student_info);
printf("Create student information successfully.\n");
break;
}
case 2: {
int id, age;
char name[20];
printf("Please enter the student information:\n");
printf("ID: ");
scanf("%d", &id);
printf("Name: ");
scanf("%s", name);
printf("Age: ");
scanf("%d", &age);
StudentInfo *new_student_info = create_student_info(id, name, age);
head = add_student_info(head, new_student_info);
printf("Input student information successfully.\n");
break;
}
case 3: {
int id;
printf("Please enter the ID of the student to be queried: ");
scanf("%d", &id);
StudentInfo *student_info = find_student_info(head, id);
if (student_info == NULL) {
printf("There is no student information with ID %d.\n", id);
} else {
print_student_info(student_info);
}
break;
}
case 4: {
int id;
printf("Please enter the ID of the student to be modified: ");
scanf("%d", &id);
StudentInfo *student_info = find_student_info(head, id);
if (student_info == NULL) {
printf("There is no student information with ID %d.\n", id);
} else {
modify_student_info(student_info);
printf("Modify student information with ID %d successfully.\n", id);
}
break;
}
case 5: {
int id;
printf("Please enter the ID of the student to be deleted: ");
scanf("%d", &id);
head = delete_student_info(head, id);
break;
}
case 6: {
print_all_student_info(head);
break;
}
case 7: {
char filename[50];
printf("Please enter the name of the file to save student information: ");
scanf("%s", filename);
save_student_info_to_file(head, filename);
break;
}
case 8: {
char filename[50];
printf("Please enter the name of the file to load student information: ");
scanf("%s", filename);
head = load_student_info_from_file(head, filename);
break;
}
case 9: {
sort_student_info(head, compare_by_id);
printf("Sort student information by ID successfully.\n");
break;
}
case 10: {
sort_student_info(head, compare_by_name);
printf("Sort student information by name successfully.\n");
break;
}
default:
printf("Invalid choice.\n");
}
}
return 0;
}
```
该程序使用单链表存储学生信息,具有创建、输入、查询、修改、删除、输出、保存到文件、从文件加载等功能,并且实现了按ID和姓名排序的功能。您可以根据需要对程序进行修改和扩展。
阅读全文
相关推荐
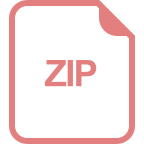

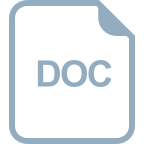
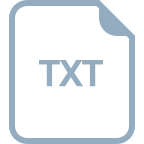
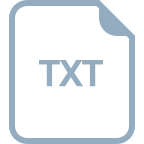
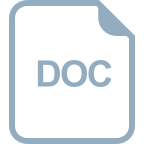
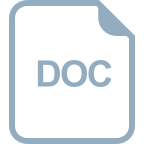
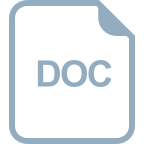
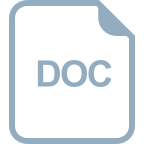
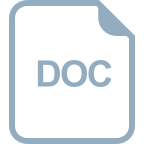
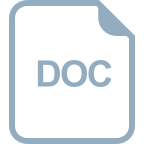
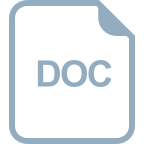
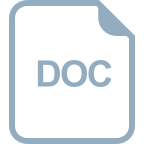
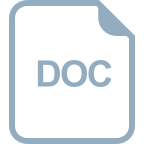
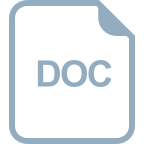
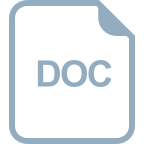