用汇编语言写一个计算器
时间: 2023-08-14 12:06:29 浏览: 40
好的,下面是一个简单的计算器汇编代码,它可以进行加、减、乘、除四种基本运算:
```assembly
section .data
prompt db "Enter an operation (+, -, *, /): ", 0
num1_prompt db "Enter the first number: ", 0
num2_prompt db "Enter the second number: ", 0
result_prompt db "Result: ", 0
error_msg db "Error: division by zero", 0
section .bss
num1 resb 2
num2 resb 2
result resb 2
section .text
global _start
_start:
; print prompt for operation
mov eax, 4
mov ebx, 1
mov ecx, prompt
mov edx, 30
int 0x80
; read operation from user input
mov eax, 3
mov ebx, 0
mov ecx, num1
mov edx, 2
int 0x80
; print prompt for first number
mov eax, 4
mov ebx, 1
mov ecx, num1_prompt
mov edx, 20
int 0x80
; read first number from user input
mov eax, 3
mov ebx, 0
mov ecx, num1
mov edx, 2
int 0x80
; print prompt for second number
mov eax, 4
mov ebx, 1
mov ecx, num2_prompt
mov edx, 21
int 0x80
; read second number from user input
mov eax, 3
mov ebx, 0
mov ecx, num2
mov edx, 2
int 0x80
; convert numbers from ASCII to binary
mov al, [num1]
sub al, 0x30
mov bl, [num2]
sub bl, 0x30
; perform operation
cmp byte [num1], '+'
je add_numbers
cmp byte [num1], '-'
je subtract_numbers
cmp byte [num1], '*'
je multiply_numbers
cmp byte [num1], '/'
je divide_numbers
; handle invalid operation
mov eax, 4
mov ebx, 1
mov ecx, error_msg
mov edx, 21
int 0x80
jmp exit_program
add_numbers:
add al, bl
jmp print_result
subtract_numbers:
sub al, bl
jmp print_result
multiply_numbers:
mul bl
jmp print_result
divide_numbers:
cmp bl, 0
je division_by_zero
div bl
jmp print_result
division_by_zero:
; handle division by zero
mov eax, 4
mov ebx, 1
mov ecx, error_msg
mov edx, 21
int 0x80
jmp exit_program
print_result:
; convert result from binary to ASCII
add al, 0x30
mov [result], al
; print result
mov eax, 4
mov ebx, 1
mov ecx, result_prompt
mov edx, 8
int 0x80
mov eax, 4
mov ebx, 1
mov ecx, result
mov edx, 2
int 0x80
exit_program:
; exit program
mov eax, 1
xor ebx, ebx
int 0x80
```
这段代码使用了 `int 0x80` 作为系统调用来读取用户输入和输出结果。该计算器支持的数字位数为两位,如果需要扩展,需要修改 `.bss` 段和相关代码。
阅读全文
相关推荐




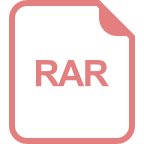
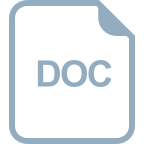

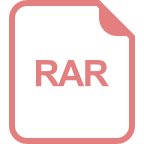

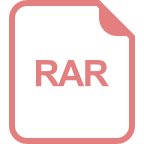
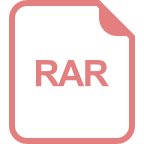






