使用yolov5定位车牌的python代码
时间: 2023-10-24 19:10:52 浏览: 47
以下是使用 YOLOv5 定位车牌的 Python 代码示例:
```python
import cv2
import numpy as np
import argparse
# 定义命令行参数
ap = argparse.ArgumentParser()
ap.add_argument("-i", "--image", required=True, help="input image path")
ap.add_argument("-w", "--weights", required=True, help="path to weights file")
ap.add_argument("-c", "--confidence", type=float, default=0.5, help="minimum confidence for detection")
ap.add_argument("-t", "--threshold", type=float, default=0.3, help="threshold when applying non-maxima suppression")
args = vars(ap.parse_args())
# 加载模型
net = cv2.dnn.readNetFromDarknet("yolov5.cfg", args["weights"])
output_layer_names = net.getLayerNames()
output_layer_names = [output_layer_names[i[0] - 1] for i in net.getUnconnectedOutLayers()]
# 加载图像
image = cv2.imread(args["image"])
(H, W) = image.shape[:2]
# 预处理图像
blob = cv2.dnn.blobFromImage(image, 1 / 255.0, (416, 416), swapRB=True, crop=False)
# 将图像输入到网络中进行检测
net.setInput(blob)
layer_outputs = net.forward(output_layer_names)
# 初始化边界框、置信度和类别ID列表
boxes = []
confidences = []
class_ids = []
# 遍历每个输出层
for output in layer_outputs:
# 遍历每个检测
for detection in output:
# 提取类别ID和置信度
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
# 过滤掉置信度低于阈值的检测
if confidence > args["confidence"]:
# 计算边界框的坐标
box = detection[0:4] * np.array([W, H, W, H])
(center_x, center_y, width, height) = box.astype("int")
# 计算边界框的左上角坐标
x = int(center_x - (width / 2))
y = int(center_y - (height / 2))
# 更新边界框、置信度和类别ID列表
boxes.append([x, y, int(width), int(height)])
confidences.append(float(confidence))
class_ids.append(class_id)
# 应用非最大值抑制
indices = cv2.dnn.NMSBoxes(boxes, confidences, args["confidence"], args["threshold"])
# 遍历每个检测
for i in indices.flatten():
# 提取边界框的坐标和尺寸
(x, y) = (boxes[i][0], boxes[i][1])
(w, h) = (boxes[i][2], boxes[i][3])
# 绘制边界框和类别标签
color = [int(c) for c in COLORS[class_ids[i]]]
cv2.rectangle(image, (x, y), (x + w, y + h), color, 2)
text = "{}: {:.4f}".format(CLASSES[class_ids[i]], confidences[i])
cv2.putText(image, text, (x, y - 5), cv2.FONT_HERSHEY_SIMPLEX, 0.5, color, 2)
# 显示输出图像
cv2.imshow("Output", image)
cv2.waitKey(0)
```
需要注意的是,以上代码中使用的是 YOLOv5 的 Darknet 版本,因此需要使用对应的配置文件 `yolov5.cfg`。此外,还需要定义类别标签和颜色列表。你需要根据自己的需求修改代码和配置文件。
相关推荐
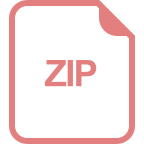
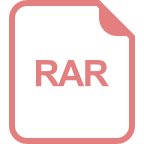














