你现在是前端工程师你要开发一个可拖拽的前端流程图,代码写出来
时间: 2023-12-10 22:39:22 浏览: 116
好的,我可以给你提供一个简单的示例代码,使用了HTML5中的`drag`和`drop`事件,可以实现拖拽节点和连接两个节点的功能。代码如下:
```html
<!DOCTYPE html>
<html>
<head>
<title>Flowchart</title>
<style>
.box {
width: 150px;
height: 100px;
background-color: #ccc;
border: 1px solid #000;
text-align: center;
line-height: 100px;
}
.connection {
position: absolute;
border: 2px solid #000;
pointer-events: none;
}
</style>
</head>
<body>
<h1>Flowchart</h1>
<div class="box" id="box1" draggable="true" ondragstart="drag(event)">Node 1</div>
<div class="box" id="box2" draggable="true" ondragstart="drag(event)">Node 2</div>
<<div class="box" id="box3" draggable="true" ondragstart="drag(event)">Node 3</div>
<script>
var connections = [];
function drag(event) {
event.dataTransfer.setData('text/plain', event.target.id);
}
function allowDrop(event) {
event.preventDefault();
}
function drop(event) {
event.preventDefault();
var sourceId = event.dataTransfer.getData('text/plain');
var targetId = event.target.id;
if (sourceId != targetId && !isConnected(sourceId, targetId)) {
var connection = drawConnection(sourceId, targetId);
document.body.appendChild(connection);
connections.push({
source: sourceId,
target: targetId,
connection: connection
});
}
}
function isConnected(sourceId, targetId) {
for (var i = 0; i < connections.length; i++) {
if ((connections[i].source == sourceId && connections[i].target == targetId) ||
(connections[i].source == targetId && connections[i].target == sourceId)) {
return true;
}
}
return false;
}
function drawConnection(sourceId, targetId) {
var source = document.getElementById(sourceId);
var target = document.getElementById(targetId);
var connection = document.createElement('div');
connection.className = 'connection';
connection.style.left = Math.min(source.offsetLeft, target.offsetLeft) + source.offsetWidth + 'px';
connection.style.top = Math.min(source.offsetTop, target.offsetTop) + source.offsetHeight / 2 - 1 + 'px';
connection.style.width = Math.abs(source.offsetLeft - target.offsetLeft) + 'px';
connection.style.height = '2px';
return connection;
}
</script>
</body>
</html>
```
这个示例代码中,我们定义了三个可拖拽的节点,每个节点都有一个`id`属性用来标识它们。当用户拖动节点时,会触发`drag`事件,我们在这个事件处理函数中使用`event.dataTransfer.setData`方法将节点的`id`信息保存到`dataTransfer`对象中,以便在`drop`事件中获取。
当用户将节点拖到另一个节点上方并释放鼠标时,会触发`drop`事件,我们在这个事件处理函数中首先使用`event.preventDefault`方法阻止浏览器默认的拖放行为。然后获取源节点和目标节点的`id`信息,并检查它们是否已经连接过,如果没有连接过,则调用`drawConnection`函数绘制连接线,并将连接信息保存到`connections`数组中。
`drawConnection`函数会创建一个`div`元素用来表示连接线,该元素使用了`position: absolute`属性,以便在页面上自由定位。我们通过计算源节点和目标节点之间的距离和位置来计算连接线的位置和大小,并将其设置为`connection`元素的`style`属性,最后返回这个元素。
如果你想让这个示例代码更加完整和实用,你可以添加更多的节点、调整节点和连接线的样式、支持删除连接等功能,但这需要更多的代码和技巧来实现。
阅读全文
相关推荐
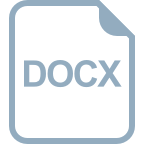
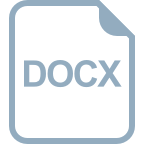
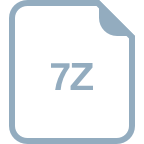
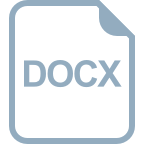
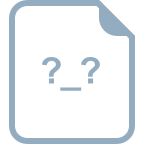
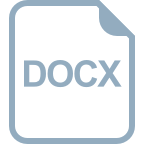
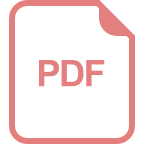
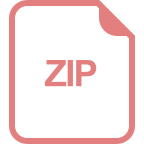
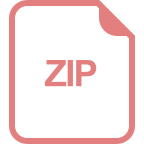
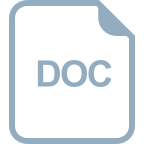
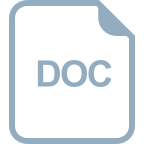
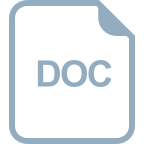
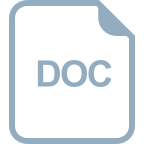
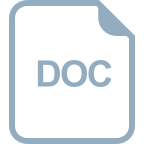
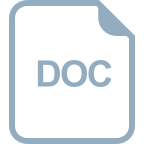
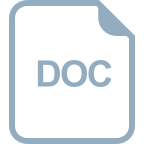
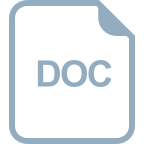