@Around("execution(void com.itheima.service.impl.DeptServiceImpl.findAll())") public Object m2(ProceedingJoinPoint pjp) { Object obj = null; //前置通知 System.out.println("1.进入目标方法之前执行"); try { //执行被代理的方法 obj = pjp.proceed(); //后置通知 System.out.println("2.执行目标方法获取返回值之后"); } catch (Throwable e) { //异常通知 System.out.println("3.方法抛出异常之后执行"); } finally { // 最终通知 System.out.println("4.最终通知:无论是否抛出异常都会执行(finally)"); } return obj; }运行流程及原理详解
时间: 2024-02-15 21:29:07 浏览: 99
这段代码是一个AOP(面向切面编程)的示例。它定义了一个切面类,使用了Spring AOP框架的注解来定义切点和通知类型。
具体运行流程如下:
1. 在DeptServiceImpl的findAll方法执行前,AOP框架会先执行m2方法中的前置通知,即输出“1.进入目标方法之前执行”。
2. 然后,AOP框架会调用被代理的findAll方法,获取返回值。
3. 在findAll方法执行完毕后,AOP框架会执行m2方法中的后置通知,即输出“2.执行目标方法获取返回值之后”。
4. 如果findAll方法执行过程中发生了异常,AOP框架会执行m2方法中的异常通知,即输出“3.方法抛出异常之后执行”。
5. 无论findAll方法是否抛出异常,AOP框架都会执行m2方法中的最终通知,即输出“4.最终通知:无论是否抛出异常都会执行(finally)”。
这种方式可以实现对目标方法的拦截和增强,比如在方法执行前后打印日志、记录方法执行时间等。原理是使用动态代理,在运行时生成代理对象,在代理对象中插入切面逻辑,从而实现对目标方法的拦截和增强。
相关问题
@Pointcut("execution(* com.itheima.service.impl. *.*(..))")中的*.*是什么意思
在 @Pointcut 表达式中,"*.*" 表示匹配任意类的任意方法,其中第一个 "*" 表示匹配任意类,第二个 "*" 表示匹配任意方法。因此,这个切入点表达式会匹配 com.itheima.service.impl 包中的任意类的任意方法。如果你想要匹配某个具体的类或方法,可以在 "*" 中指定类名或方法名。例如,"execution(* com.itheima.service.impl.UserServiceImpl.*(..))" 表示匹配 com.itheima.service.impl 包中的 UserServiceImpl 类的所有方法。
org.aspectj.lang.ProceedingJoinPoint
`org.aspectj.lang.ProceedingJoinPoint`是AspectJ框架中的一个重要类,它代表在程序执行期间切面所织入的连接点。当在AspectJ切面中声明了一个带有`ProceedingJoinPoint`类型参数的方法,该方法会在连接点处执行。通过`ProceedingJoinPoint`对象,切面可以获取连接点上下文中的信息,例如方法参数、目标对象和执行方法所需的参数等。下面是一个例子,演示了如何使用`ProceedingJoinPoint`来获取方法参数并执行方法:
<<引用:import org.aspectj.lang.ProceedingJoinPoint;import org.aspectj.lang.annotation.Around;import org.aspectj.lang.annotation.Aspect;import org.aspectj.lang.annotation.Pointcut;@Aspectpublic class LoggingAspect {@Pointcut("execution(* com.example.myapp.service.*.*(..))")private void serviceMethods() {}@Around("serviceMethods()")public Object logAround(ProceedingJoinPoint joinPoint) throws Throwable { // 获取方法参数Object[] args = joinPoint.getArgs(); // 执行方法Object result = joinPoint.proceed(); return result;}}[^1]>>
阅读全文
相关推荐



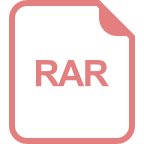


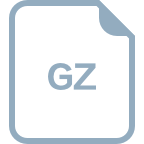




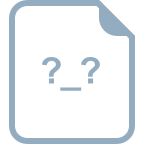

