mybatisplus查询数据库中一张表的前100条数据,如何写
时间: 2024-05-06 10:15:09 浏览: 9
使用MyBatis Plus查询数据库中一张表的前100条数据,可以按照以下步骤进行操作:
1. 导入MyBatis Plus依赖,例如:
```
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.x.x</version>
</dependency>
```
2. 创建对应的实体类,并使用`@TableName`注解指定对应的表名,例如:
```
import com.baomidou.mybatisplus.annotation.TableName;
@TableName("user")
public class User {
private Long id;
private String name;
private Integer age;
// getter/setter 略
}
```
3. 创建对应的Mapper接口,并继承`BaseMapper`接口,例如:
```
import com.baomidou.mybatisplus.core.mapper.BaseMapper;
public interface UserMapper extends BaseMapper<User> {
}
```
4. 在对应的Service中使用`BaseMapper`提供的方法进行查询,例如:
```
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class UserService {
@Autowired
private UserMapper userMapper;
public List<User> getTop100Users() {
QueryWrapper<User> wrapper = new QueryWrapper<>();
wrapper.last("limit 100");
return userMapper.selectList(wrapper);
}
}
```
在上述代码中,`QueryWrapper`是MyBatis Plus提供的查询条件构造器,`last`方法可以在SQL语句的最后添加任意字符串,这里添加了`limit 100`来限制查询结果的数量。最后通过调用`selectList`方法来执行查询并返回查询结果。
这样就可以使用MyBatis Plus查询数据库中一张表的前100条数据了。
相关推荐
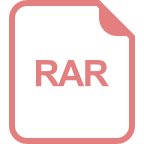
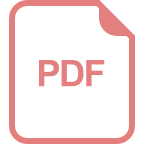
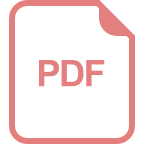








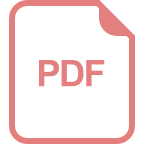
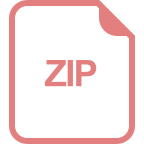
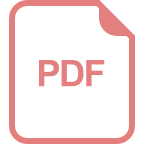
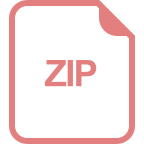
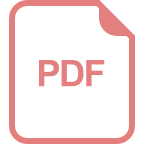
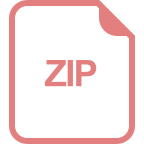