vue3怎么同时定义三个定时器分别为10秒,20秒,30秒,前端根据弹窗页面选择后点击执行按钮执行对应定时器或点击取消按钮不执行,使用ref方法和script setup里面编写,功能在子组件中,弹窗在父组件中,并且项目中使用了element -ui的组件
时间: 2024-03-03 18:50:51 浏览: 20
可以使用`ref`和`watch`来实现此功能。首先在子组件中定义三个`ref`变量分别表示三个定时器:
```
<template>
<div></div>
</template>
<script setup>
import { ref } from 'vue';
const timer1 = ref(null);
const timer2 = ref(null);
const timer3 = ref(null);
function startTimer() {
timer1.value = setTimeout(() => {
// 10秒后执行的代码
}, 10000);
timer2.value = setTimeout(() => {
// 20秒后执行的代码
}, 20000);
timer3.value = setTimeout(() => {
// 30秒后执行的代码
}, 30000);
}
function stopTimer() {
clearTimeout(timer1.value);
clearTimeout(timer2.value);
clearTimeout(timer3.value);
}
</script>
```
然后在父组件中引入子组件并使用`el-dialog`组件来实现弹窗。在弹窗中添加两个按钮,一个是执行按钮,一个是取消按钮。点击执行按钮时,根据用户选择的定时器来执行对应的定时器函数。点击取消按钮时,调用子组件中的停止定时器函数:
```
<template>
<div>
<el-button @click="dialogVisible = true">弹窗</el-button>
<el-dialog
title="选择定时器"
:visible.sync="dialogVisible"
width="30%"
:before-close="beforeClose"
>
<el-radio-group v-model="selectedTimer">
<el-radio-button label="1">10秒</el-radio-button>
<el-radio-button label="2">20秒</el-radio-button>
<el-radio-button label="3">30秒</el-radio-button>
</el-radio-group>
<div style="text-align: right; margin: 20px 0;">
<el-button @click="execute">执行</el-button>
<el-button @click="cancel">取消</el-button>
</div>
</el-dialog>
<child-component ref="child"></child-component>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent,
},
data() {
return {
dialogVisible: false,
selectedTimer: '',
};
},
methods: {
beforeClose(done) {
this.dialogVisible = false;
this.selectedTimer = '';
done();
},
execute() {
if (this.selectedTimer === '1') {
this.$refs.child.timer1.value();
} else if (this.selectedTimer === '2') {
this.$refs.child.timer2.value();
} else if (this.selectedTimer === '3') {
this.$refs.child.timer3.value();
}
this.dialogVisible = false;
this.selectedTimer = '';
},
cancel() {
this.$refs.child.stopTimer();
this.dialogVisible = false;
this.selectedTimer = '';
},
},
};
</script>
```
在父组件的`execute`方法中,根据用户选择的定时器来执行对应的定时器函数。在取消按钮中,调用子组件的停止定时器函数来停止所有定时器。
相关推荐
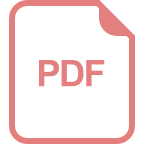















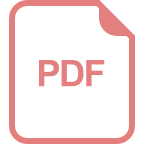