springboot的配置类的示例和实体类的示例和接口的示例
时间: 2024-03-23 09:37:44 浏览: 57
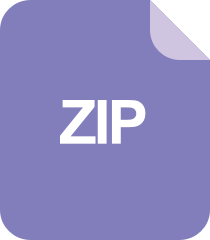
springboot 实例

好的,下面是Spring Boot的配置类示例、实体类示例和接口示例。
**配置类示例**
```java
@Configuration
public class AppConfig {
@Bean
public SomeService someService() {
return new SomeServiceImpl();
}
@Bean
public AnotherService anotherService() {
return new AnotherServiceImpl(someService());
}
}
```
上面的示例展示了如何使用@Configuration注释创建一个配置类。该类包含了两个@Bean方法,用于声明和创建SomeService和AnotherService的实例。其中,AnotherService需要依赖SomeService,因此我们在创建AnotherService实例时,通过someService()方法获取SomeService的实例。
**实体类示例**
```java
@Entity
@Table(name = "students")
public class Student {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "name")
private String name;
@Column(name = "age")
private int age;
// 省略getter和setter方法
}
```
上面的示例展示了如何使用@Entity注释创建一个实体类。该类会被映射到数据库中的students表,每个字段会被映射到对应的表列中。其中,@Id注释表示该字段为主键,@GeneratedValue注释表示该字段的值由数据库自动生成。
**接口示例**
```java
@RestController
@RequestMapping("/students")
public class StudentController {
@Autowired
private StudentService studentService;
@GetMapping("/{id}")
public Student getStudent(@PathVariable Long id) {
return studentService.getStudentById(id);
}
@PostMapping
public Student createStudent(@RequestBody Student student) {
return studentService.createStudent(student);
}
// 省略其他方法
}
```
上面的示例展示了如何使用@RestController注释创建一个RESTful风格的接口类。该类包含了两个方法,用于获取和创建Student实例。其中,@GetMapping和@PostMapping注释分别表示使用HTTP GET和POST方法访问该方法,@PathVariable注释表示获取URL中的参数,@RequestBody注释表示获取请求体中的参数。同时,我们使用@Autowired注释自动注入了一个StudentService实例,用于处理业务逻辑。
阅读全文
相关推荐
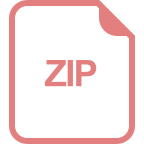
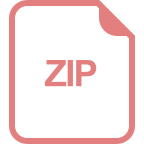
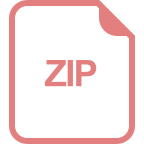
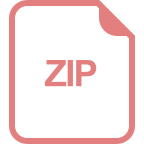
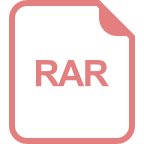
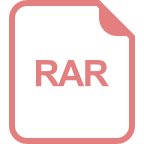
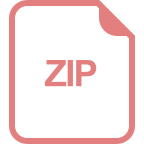
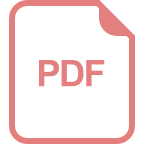
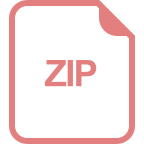
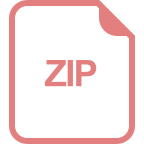
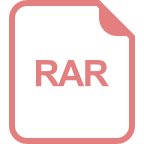
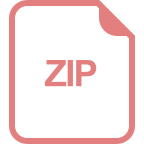
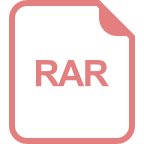
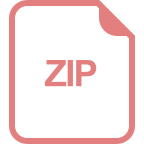
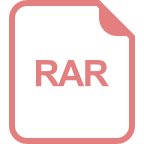