tensorflow 加载本地物体识别数据集
时间: 2023-07-29 20:13:41 浏览: 53
要在 TensorFlow 中加载本地物体识别数据集,可以使用 TensorFlow 的数据集 API。以下是一些步骤:
1. 准备数据集:将数据集组织为文件夹结构,每个文件夹包含一个类别的图像。例如,如果数据集有两个类别(猫和狗),则可以创建两个文件夹(cat 和 dog),并在每个文件夹中放置相应类别的图像。
2. 使用 `tf.keras.preprocessing.image_dataset_from_directory` 函数加载数据集。该函数将图像自动调整为统一的大小,并将其转换为 TensorFlow 的 `tf.data.Dataset` 对象。以下是一个示例代码片段:
```
import tensorflow as tf
# 定义数据集路径和图像大小
data_dir = '/path/to/dataset'
img_size = (224, 224)
# 使用 tf.keras.preprocessing.image_dataset_from_directory 函数加载数据集
ds_train = tf.keras.preprocessing.image_dataset_from_directory(
data_dir,
validation_split=0.2,
subset='training',
seed=123,
image_size=img_size,
batch_size=32)
```
3. 对数据集进行预处理。可以使用 TensorFlow 的 `tf.data` API 中的各种转换和操作来对数据集进行预处理。例如,可以使用 `map` 方法应用图像增强操作,或使用 `cache` 方法缓存数据集以提高性能。以下是一个示例代码片段:
```
# 对数据集进行预处理
ds_train = ds_train.map(
lambda x, y: (tf.image.resize(x, img_size), y))
ds_train = ds_train.cache()
ds_train = ds_train.shuffle(ds_train.cardinality().numpy())
ds_train = ds_train.prefetch(tf.data.experimental.AUTOTUNE)
```
4. 构建模型:可以使用 TensorFlow 的 Keras API 来构建模型。以下是一个示例代码片段:
```
# 构建模型
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, 3, activation='relu', input_shape=(224, 224, 3)),
tf.keras.layers.MaxPooling2D(),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(2, activation='softmax')
])
```
5. 训练模型:使用 TensorFlow 的 Keras API 中的 `fit` 方法来训练模型。以下是一个示例代码片段:
```
# 训练模型
model.compile(
optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
model.fit(ds_train, epochs=10)
```
这些步骤可以帮助你在 TensorFlow 中加载本地物体识别数据集并训练模型。
相关推荐
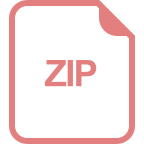














