python实现删除某个文件夹的单个txt文件
时间: 2024-05-12 13:17:54 浏览: 7
可以使用Python的os模块和os.path模块来实现删除某个文件夹中的单个txt文件。具体代码如下:
```
import os
folder_path = "your_folder_path"
file_name = "your_file_name.txt"
file_path = os.path.join(folder_path, file_name)
if os.path.exists(file_path):
os.remove(file_path)
print(f"{file_name} has been deleted!")
else:
print(f"{file_name} does not exist!")
```
其中,`folder_path`为文件夹路径,`file_name`为要删除的文件名。使用`os.path.join()`函数可以将文件夹路径和文件名组合成完整的文件路径。使用`os.path.exists()`函数可以判断文件是否存在,如果存在,则使用`os.remove()`函数删除文件,否则输出提示信息。
相关问题
python实现删除某个文件夹的txt文件
您可以使用Python中的os模块和shutil模块来删除某个文件夹中的txt文件。以下是一个示例代码:
```python
import os
import shutil
folder_path = "/path/to/folder"
for filename in os.listdir(folder_path):
if filename.endswith(".txt"):
file_path = os.path.join(folder_path, filename)
os.remove(file_path)
```
请将`/path/to/folder`替换为您要删除txt文件的文件夹的路径。这段代码将遍历文件夹中的所有文件,并删除扩展名为`.txt`的文件。
python获取某个文件夹的所有txt文件
你可以使用Python内置的`os`和`glob`模块来实现获取某个文件夹中所有的`.txt`文件。
示例代码如下:
```python
import os
import glob
# 设置文件夹路径
folder_path = 'path/to/folder/'
# 获取所有txt文件
txt_files = glob.glob(os.path.join(folder_path, '*.txt'))
# 打印所有txt文件路径
for file_path in txt_files:
print(file_path)
```
其中,`os.path.join()`函数用于拼接文件夹路径和文件名,`glob.glob()`函数用于获取符合通配符模式的文件路径列表。上述示例代码中,`*.txt`代表所有的`.txt`文件。
相关推荐
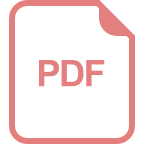
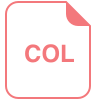











