def _make_denseblock(self, block, blocks): layers = [] for i in range(blocks): # Currently we fix the expansion ratio as the default value layers.append(block(self.inplanes, growthRate=self.growthRate, dropRate=self.dropRate)) self.inplanes += self.growthRate return nn.Sequential(*layers) 这段代码意思
时间: 2024-04-26 16:21:49 浏览: 64
这段代码实现了一个 Dense Block 的生成函数 `_make_denseblock`,它接受两个参数:`block` 表示要使用的基础网络层,`blocks` 表示要生成的 Dense Block 的个数。函数返回一个由 Dense Block 中的所有网络层按顺序组成的 PyTorch 的 `nn.Sequential` 对象。
在函数中,首先定义了一个空列表 `layers`,然后通过一个循环来生成 Dense Block 中的每个网络层。每次循环中,都会调用 `block` 函数来生成一个网络层,并将其添加到 `layers` 列表中。其中,`self.inplanes` 表示当前输入的通道数,`self.growthRate` 表示每个网络层要增加的通道数,`self.dropRate` 表示每个网络层的 dropout 概率。在每次循环结束后,都会将 `self.inplanes` 更新为当前的通道数,这样下一个网络层的输入通道数就是上一个网络层的输出通道数。
最后,函数返回一个 `nn.Sequential` 对象,其中包含了所有生成的网络层。这个对象可以被视为一个整体,方便在后续的神经网络模型中使用。
相关问题
def _make_denseblock(self, block, blocks): layers = [] for i in range(blocks): # Currently we fix the expansion ratio as the default value layers.append(block(self.inplanes, growthRate=self.growthRate, dropRate=self.dropRate)) self.inplanes += self.growthRate return nn.Sequential(*layers) def _make_transition(self, compressionRate): inplanes = self.inplanes outplanes = int(math.floor(self.inplanes // compressionRate)) self.inplanes = outplanes return transition_2D(inplanes, outplanes)
这段代码看起来像是一个 PyTorch 的神经网络模型的定义,其中包括了 `_make_denseblock` 和 `_make_transition` 两个函数。`_make_denseblock` 函数的作用是生成若干个 Dense Block,每个 Dense Block 中包含了若干个相同的神经网络层,每个神经网络层的输入都是前面所有层的输出的拼接,这样可以使得信息传递更加充分。`_make_transition` 函数则是生成 DenseNet 中的过渡层,将神经网络层数较多的 Dense Block 的输出压缩到一个更小的维度,以减少参数数量和计算量。具体实现细节可以参考代码中调用的 `block` 和 `transition_2D` 函数的定义。
#256个block memory_size = 256 #pid进程号 class Process: def __init__(self, pid, block, duration): self.__block = block self.__duration = duration self.__pid = pid self.__memory = None @property def pid(self): return self.__pid @property def block(self): return self.__block @property def duration(self): return self.__duration def set_memory(self, memory_start, memory_end): self.__memory = (memory_start, memory_end) def get_memory(self): return self.__memory class MemoryAllocator: def __init__(self, memory_size): self.__memory_blocks = [None] * memory_size def memory_view(self): '''return the array of the use of memory blocks.''' return tuple(self.__memory_blocks) def allocate_memory(self, block_start, length, process): for block_id in range(block_start, block_start+length): assert self.__memory_blocks[block_id] is None, 'tend to allocate occupied blocks' self.__memory_blocks[block_id] = process process.set_memory(block_start, length) def free_memory(self, process): assert process.get_memory() is not None, 'process should already hold memory blocks' block_start, length = process.get_memory() for block_id in range(block_start, block_start+length): assert self.__memory_blocks[block_id] == process, 'the orresponding memory blocks should be assigned to the process' self.__memory_blocks[block_id] = None
这段代码实现了一个简单的内存分配器和进程类。其中,内存分配器使用一个数组来表示内存块的使用情况,每个进程对象都包含一个指向内存块的引用。
这段代码的实现还可以进行一些改进:
1. 内存分配器可以使用更高效的数据结构,比如位图,来表示内存块的使用情况。
2. 进程类可以添加一个方法来释放内存,而不是在内存分配器中实现。
3. 内存分配器可以添加一个方法来查找可用的内存块,而不是在进程类中实现。
4. 进程类可以将内存块的起始位置和长度作为属性,而不是在set_memory方法中设置。
下面是改进后的代码:
```
class Process:
def __init__(self, pid, duration):
self.__pid = pid
self.__duration = duration
self.__memory_start = None
self.__memory_length = None
@property
def pid(self):
return self.__pid
@property
def duration(self):
return self.__duration
@property
def memory_start(self):
return self.__memory_start
@property
def memory_length(self):
return self.__memory_length
def set_memory(self, start, length):
self.__memory_start = start
self.__memory_length = length
def release_memory(self):
self.__memory_start = None
self.__memory_length = None
class MemoryAllocator:
def __init__(self, memory_size):
self.__memory_blocks = [False] * memory_size
def find_free_memory(self, length):
for i in range(len(self.__memory_blocks) - length + 1):
if all(not self.__memory_blocks[j] for j in range(i, i + length)):
return i
return None
def allocate_memory(self, process):
length = process.duration
start = self.find_free_memory(length)
assert start is not None, 'allocation failed'
for i in range(start, start + length):
self.__memory_blocks[i] = True
process.set_memory(start, length)
def free_memory(self, process):
start = process.memory_start
length = process.memory_length
for i in range(start, start + length):
self.__memory_blocks[i] = False
process.release_memory()
```
改进后的代码更加清晰和易于维护,也更加高效。
阅读全文
相关推荐

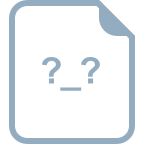














