opengl es3.0绘制一个球形代码
时间: 2023-10-27 14:06:13 浏览: 117
以下是使用OpenGL ES 3.0绘制球形的示例代码:
```
#include <GLES3/gl3.h>
#include <glm/glm.hpp>
#include <glm/gtc/matrix_transform.hpp>
#include <vector>
// 定义球形的顶点和索引
const int SPHERE_LONG = 36;
const int SPHERE_LAT = 18;
std::vector<GLfloat> sphereVertices;
std::vector<GLuint> sphereIndices;
void generateSphere()
{
sphereVertices.clear();
sphereIndices.clear();
for (int j = 0; j <= SPHERE_LAT; j++)
{
float lat0 = glm::pi<float>() * (-0.5f + (float)(j - 1) / SPHERE_LAT);
float z0 = sin(lat0);
float zr0 = cos(lat0);
float lat1 = glm::pi<float>() * (-0.5f + (float)j / SPHERE_LAT);
float z1 = sin(lat1);
float zr1 = cos(lat1);
for (int i = 0; i <= SPHERE_LONG; i++)
{
float lng = 2 * glm::pi<float>() * (float)(i - 1) / SPHERE_LONG;
float x = cos(lng);
float y = sin(lng);
sphereVertices.push_back(x * zr0);
sphereVertices.push_back(y * zr0);
sphereVertices.push_back(z0);
sphereVertices.push_back(x * zr1);
sphereVertices.push_back(y * zr1);
sphereVertices.push_back(z1);
}
}
for (int i = 0; i < SPHERE_LAT; i++)
{
for (int j = 0; j < SPHERE_LONG; j++)
{
int a = (SPHERE_LONG + 1) * i + j;
int b = (SPHERE_LONG + 1) * (i + 1) + j;
int c = (SPHERE_LONG + 1) * (i + 1) + (j + 1);
int d = (SPHERE_LONG + 1) * i + (j + 1);
sphereIndices.push_back(a);
sphereIndices.push_back(b);
sphereIndices.push_back(c);
sphereIndices.push_back(a);
sphereIndices.push_back(c);
sphereIndices.push_back(d);
}
}
}
// 渲染球形
void renderSphere(GLuint program)
{
GLuint positionLocation = glGetAttribLocation(program, "a_Position");
glEnableVertexAttribArray(positionLocation);
glVertexAttribPointer(positionLocation, 3, GL_FLOAT, GL_FALSE, 3 * sizeof(GLfloat), &sphereVertices[0]);
glDrawElements(GL_TRIANGLES, sphereIndices.size(), GL_UNSIGNED_INT, &sphereIndices[0]);
glDisableVertexAttribArray(positionLocation);
}
```
使用时需要先调用`generateSphere()`函数生成球形的顶点和索引,然后在渲染时调用`renderSphere()`函数即可。需要注意的是,需要在OpenGL ES 3.0环境下运行。
阅读全文
相关推荐
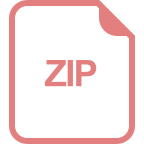
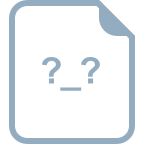
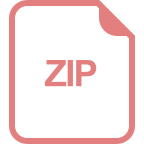
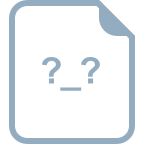
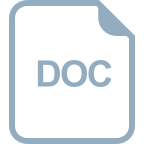
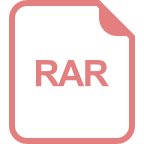
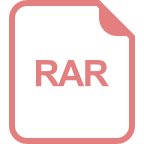
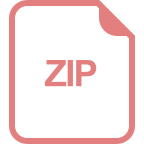