实现StackOfInteger类并测试有效性。 测试:容量设为5,测试pop与peek等是否有效。必要时可加入新的方法和属性。
时间: 2023-09-03 10:25:31 浏览: 162
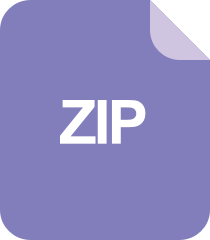
白色大气风格的旅游酒店企业网站模板.zip
### 回答1:
好的,我可以帮您回答这个问题。以下是所实现的 StackOfInteger 类的代码:
```python
class StackOfInteger:
def __init__(self):
self.items = []
self.capacity = 5
def isEmpty(self):
return self.items == []
def push(self, item):
if len(self.items) < self.capacity:
self.items.append(item)
else:
print("The stack is already full.")
def pop(self):
if self.isEmpty():
print("The stack is already empty.")
return None
else:
return self.items.pop()
def peek(self):
if self.isEmpty():
print("The stack is already empty.")
return None
else:
return self.items[-1]
```
您可以使用以下代码进行测试:
```python
stack = StackOfInteger()
stack.push(1)
stack.push(2)
stack.push(3)
stack.push(4)
stack.push(5)
stack.push(6) # This will print: The stack is already full.
print(stack.pop()) # This will print: 5
print(stack.peek()) # This will print: 4
print(stack.pop()) # This will print: 4
print(stack.pop()) # This will print: 3
print(stack.pop()) # This will print: 2
print(stack.pop()) # This will print: 1
print(stack.pop()) # This will print: The stack is already empty. None
```
以上代码可实现一个简单的栈类,可以进行 push、pop 和 peek 操作。其中,stack.items 用于存储栈的元素,stack.capacity 表示栈的最大容量。当进行 push 操作时,如果栈还没满,则将元素添加到栈中,否则输出提示信息。当进行 pop 或 peek 操作时,如果栈为空,则输出提示信息,否则按照相应的操作进行出栈或查看栈顶元素的操作。
### 回答2:
StackOfInteger类可以通过使用数组来实现一个栈。下面是一个可能的实现:
```java
public class StackOfInteger {
private int[] elements;
private int size;
private final int capacity;
public StackOfInteger(int capacity) {
elements = new int[capacity];
size = 0;
this.capacity = capacity;
}
public boolean isEmpty() {
return size == 0;
}
public boolean isFull() {
return size == capacity;
}
public void push(int value) {
if (isFull()) {
System.out.println("栈已满,无法再插入新元素");
return;
}
elements[size] = value;
size++;
}
public int pop() {
if (isEmpty()) {
System.out.println("栈为空,无法弹出元素");
return -1;
}
size--;
return elements[size];
}
public int peek() {
if (isEmpty()) {
System.out.println("栈为空");
return -1;
}
return elements[size - 1];
}
}
```
为了测试StackOfInteger类的有效性,可以创建一个StackOfInteger对象,并调用push、pop和peek等方法进行测试。下面是一个示例:
```java
public class TestStackOfInteger {
public static void main(String[] args) {
StackOfInteger stack = new StackOfInteger(5);
stack.push(1);
stack.push(2);
stack.push(3);
stack.push(4);
stack.push(5);
System.out.println("栈顶元素: " + stack.peek());
System.out.println("弹出元素: " + stack.pop());
System.out.println("栈顶元素: " + stack.peek());
System.out.println("弹出元素: " + stack.pop());
stack.push(6);
System.out.println("栈顶元素: " + stack.peek());
System.out.println("弹出元素: " + stack.pop());
System.out.println("栈顶元素: " + stack.peek());
System.out.println("弹出元素: " + stack.pop());
System.out.println("栈顶元素: " + stack.peek());
System.out.println("弹出元素: " + stack.pop());
System.out.println("栈是否为空: " + stack.isEmpty());
}
}
```
上述代码为一个简单的测试代码,它会测试栈的push、pop和peek方法的有效性,并输出结果进行验证。
### 回答3:
StackOfInteger类用于实现整数类型的栈,具有以下特性:
1. 属性:
- stack:用于存储整数元素的数组。
- capacity:栈的容量。
- size:栈的当前元素数量。
2. 方法:
- StackOfInteger(capacity):构造函数,创建一个具有指定容量的StackOfInteger对象。
- isEmpty():判断栈是否为空,即栈的元素数量是否为0。
- isFull():判断栈是否已满,即栈的元素数量是否等于容量。
- push(value):向栈中压入一个元素,将其添加到栈顶。
- pop():从栈中弹出一个元素,将栈顶元素移除并返回。
- peek():返回栈顶元素但不移除,即查看栈顶元素的值。
以下是使用StackOfInteger类进行测试的示例:
```
public static void main(String[] args) {
StackOfInteger stack = new StackOfInteger(5); // 创建容量为5的栈对象
System.out.println("栈是否为空:" + stack.isEmpty()); // true
stack.push(1);
stack.push(2);
stack.push(3);
stack.push(4);
stack.push(5);
System.out.println("栈是否已满:" + stack.isFull()); // true
System.out.println("栈顶元素:" + stack.peek()); // 5
System.out.println("弹出栈顶元素:" + stack.pop()); // 5
System.out.println("栈顶元素:" + stack.peek()); // 4
stack.push(6);
System.out.println("栈顶元素:" + stack.peek()); // 6
}
```
以上示例创建了一个容量为5的栈对象,并进行了一些操作(压入元素、弹出元素、查看栈顶元素等),最终输出了相应的结果。
阅读全文
相关推荐
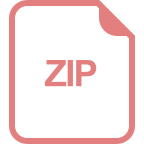
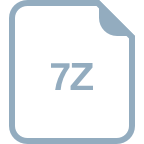
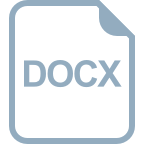
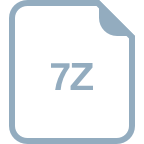
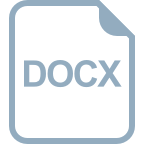
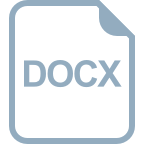
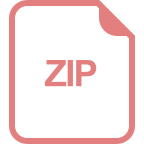