linux c语言读取配置文件的前四行行所有信息并全部保存到对应变量中
时间: 2024-02-03 16:13:46 浏览: 160
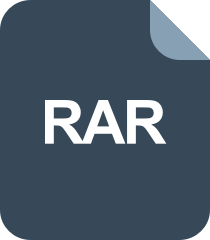
C语言实现读取配置文件程序

假设你的配置文件名为config.txt,其中前四行分别为:
```
name=LiMing
age=25
gender=male
city=Beijing
```
你可以使用以下C语言代码读取并保存这些配置信息:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LINE_LENGTH 100
int main() {
FILE *fp;
char line[MAX_LINE_LENGTH];
char name[MAX_LINE_LENGTH];
char age[MAX_LINE_LENGTH];
char gender[MAX_LINE_LENGTH];
char city[MAX_LINE_LENGTH];
int line_num = 0;
fp = fopen("config.txt", "r");
if (fp == NULL) {
printf("Failed to open config file.\n");
exit(1);
}
while (fgets(line, MAX_LINE_LENGTH, fp) != NULL && line_num < 4) {
line[strcspn(line, "\r\n")] = 0; // remove newline character
switch (line_num) {
case 0:
sscanf(line, "name=%s", name);
break;
case 1:
sscanf(line, "age=%s", age);
break;
case 2:
sscanf(line, "gender=%s", gender);
break;
case 3:
sscanf(line, "city=%s", city);
break;
}
line_num++;
}
fclose(fp);
// Print the values to verify they were correctly saved
printf("Name: %s\n", name);
printf("Age: %s\n", age);
printf("Gender: %s\n", gender);
printf("City: %s\n", city);
return 0;
}
```
该程序首先打开配置文件并逐行读取前四行,使用`fgets()`函数读取每行内容,并使用`switch`语句分别处理每行内容。对于每行,我们使用`sscanf()`函数从字符串中提取变量值,并将其保存到相应的变量中。最后,程序打印出保存在变量中的值以进行验证。值得注意的是,我们使用`strcspn()`函数删除了每行最后的换行符。
阅读全文
相关推荐
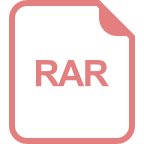
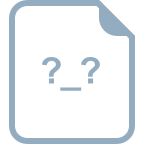
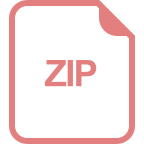
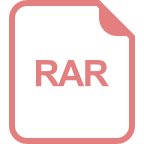
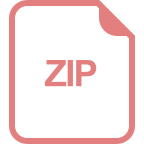
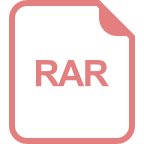
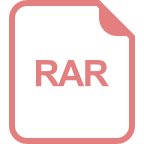
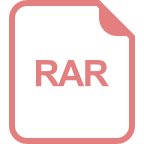
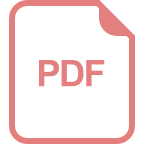
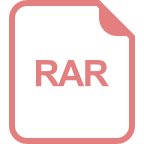
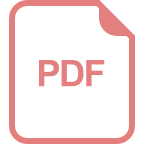