c++中atoi是什么
时间: 2024-01-31 12:02:54 浏览: 27
`atoi()` 是 C++ 标准库中的一个函数,用于将字符串转换为整数。它的函数原型如下:
```c++
int atoi(const char *str);
```
其中,`str` 参数是要转换的字符串。`atoi()` 函数会尝试将字符串解析为一个整数,并返回解析结果。如果字符串无法解析为整数,`atoi()` 函数会返回 0。
`atoi()` 函数会忽略字符串开头的空白字符,直到遇到第一个非空白字符为止。然后,它会尝试解析从该位置开始的字符序列为一个整数。如果第一个非空白字符是正负号,则 `atoi()` 函数会将其作为符号位处理。
例如,以下代码将字符串 "12345" 转换为整数:
```c++
const char *str = "12345";
int num = atoi(str);
```
在上面的代码中,`atoi()` 函数会将字符串 "12345" 解析为整数 12345,并将其存储到变量 `num` 中。
需要注意的是,`atoi()` 函数不会进行任何错误检查,如果字符串无法解析为整数,它会返回 0。因此,在实际使用中,应该使用更加健壮和安全的字符串转换函数,如 `strtol()`、`strtoul()`、`stoi()` 等。
相关问题
C++实现atoi函数
atoi函数是C语言的标准函数,用于将字符串转换为整数。在实现过程中,需要进行一些判断和转换操作。首先判断字符串长度是否大于0,然后判断首位字符的符号,再根据正负数对应的溢出条件进行判断。最后,遍历每一位字符,将对应的char类型字符转换为整数,并进行相应的运算和溢出判断。
下面是一个参考的C实现代码:
```
int my_atoi(const char* s) {
if(s == nullptr){
throw "输入异常";
}
bool negative = false;
int result = 0; // 存放中间变量
int len = my_strlen(s);
int limit = -2147483647;
int i = 0;
if(len > 0) {
if (s[0] < '0') {
if ('-' == s[0]) {
negative = true;
limit = -2147483648;
} else if (' ' != s[0]) {
throw "输入异常";
}
if(len == 1){
throw "error";
}
i++;
}
while(i < len) {
int digit = s[i] - '0';
if(digit < 0 || digit > 9) {
throw "输入异常";
} else {
result *= 10;
if(result - digit < limit) {
throw "溢出";
}
result -= digit;
}
}
} else {
cout << "error" << endl;
throw -2147483647;
}
return negative ? result : -result;
}
```
c++ atoi 原型
c++中的atoi函数用于将字符串转换为整数。它的原型如下:
```c++
int atoi(const char* str);
```
该函数接受一个指向字符串的指针作为参数,并返回相应的整数值。它会从字符串的开头开始解析,直到遇到非数字字符为止。如果字符串中的第一个字符是非数字字符,则返回0。
以下是一个使用atoi函数的示例:
```c++
#include <cstdlib>
#include <iostream>
int main() {
const char* str = "123";
int num = atoi(str);
std::cout << "Converted number: " << num << std::endl;
return 0;
}
```
该示例中,将字符串"123"转换为整数,并将结果打印出来。
相关推荐
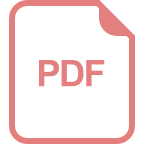
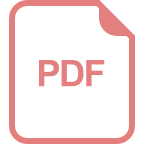












