SPD-Conv 的代码
时间: 2024-05-01 11:19:50 浏览: 89
以下是一个SPD-Conv的示例代码,用于在PyTorch中实现SPD卷积层:
```python
import torch
import torch.nn as nn
from torch.nn.parameter import Parameter
import torch.nn.functional as F
from torch.autograd import Function
class SPDConv(Function):
@staticmethod
def forward(ctx, input, weight):
ctx.save_for_backward(input, weight)
output = torch.matmul(torch.matmul(weight, input), weight.transpose(-1,-2))
return output
@staticmethod
def backward(ctx, grad_output):
input, weight = ctx.saved_tensors
grad_input = torch.matmul(torch.matmul(weight.transpose(-1,-2), grad_output), weight)
grad_weight = torch.matmul(torch.matmul(input, grad_output), weight.transpose(-1,-2))
return grad_input, grad_weight
class SPDConvLayer(nn.Module):
def __init__(self, in_channels, out_channels, kernel_size, bias=True):
super(SPDConvLayer, self).__init__()
self.in_channels = in_channels
self.out_channels = out_channels
self.kernel_size = kernel_size
self.weight = Parameter(torch.Tensor(out_channels, in_channels, kernel_size, kernel_size))
if bias:
self.bias = Parameter(torch.Tensor(out_channels))
else:
self.register_parameter('bias', None)
self.reset_parameters()
def reset_parameters(self):
nn.init.xavier_uniform_(self.weight)
if self.bias is not None:
nn.init.zeros_(self.bias)
def forward(self, input):
x = SPDConv.apply(input, self.weight)
if self.bias is not None:
x = x + self.bias.view(1, -1, 1, 1)
return x
```
在这个代码中,我们定义了一个自定义的SPDConv类,它继承了PyTorch的Function类。在前向传播中,我们调用了torch.matmul函数来计算SPD卷积的输出。在反向传播中,我们通过矩阵乘法和转置运算来计算梯度。我们还定义了一个SPDConvLayer类,它继承了PyTorch的nn.Module类,并使用我们定义的SPDConv类来实现SPD卷积层的前向传播。我们还实现了参数初始化和偏置项的添加,以方便模型的训练和使用。
阅读全文
相关推荐
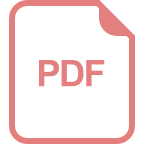
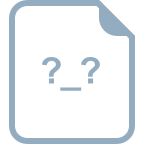
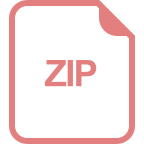
















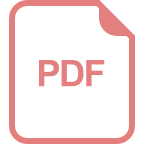