LZW编码压缩图像预期结果
时间: 2023-11-03 22:39:41 浏览: 42
LZW编码压缩图像预期结果是减小图像文件的大小,从而节省存储空间和传输时间,同时保持图像质量不受影响。LZW算法能够通过利用图像中相邻的像素的重复性和相似性,将重复的像素数据进行压缩,从而有效地减少图像文件的大小。预期结果是压缩后的图像文件大小比原始图像文件大小小,而且在解压缩后,图像质量没有明显的损失。
相关问题
lzw图像压缩编码matlab
LZW(Lempel-Ziv-Welch)压缩算法是一种无损压缩算法,能够对数据流进行有效压缩。在图像压缩方面,LZW算法可以将原始图像数据流进行压缩,并且不会损失原始图像的任何信息。下面是一个基于 MATLAB 的 LZW 图像压缩编码实现:
```matlab
function [compressedImage, dict] = lzw_compress(image)
% 将图像转换为一维数据流
data = reshape(image, 1, []);
% 初始化编码字典
dict = cell(256, 1);
for i = 1:256
dict{i} = uint8(i-1);
end
% 初始化编码参数
code = 257;
p = uint8([]);
compressedImage = [];
% LZW 编码
for i = 1:numel(data)
c = data(i);
pc = [p c];
if ismember(pc, dict)
p = pc;
else
compressedImage = [compressedImage uint16(find(ismember(dict, p)))];
dict{code} = pc;
code = code + 1;
p = c;
end
end
% 将编码后的数据流转换为字节流
compressedImage = typecast(compressedImage, 'uint8');
end
```
这个函数将输入的图像 `image` 转换为一维数据流 `data`,并且初始化编码字典。接下来,我们对数据流进行 LZW 编码,将编码后的数据转换为字节流并返回。此外,函数还返回了编码字典 `dict`,方便解码时使用。
以下是一个基于 MATLAB 的 LZW 图像解压缩编码实现:
```matlab
function image = lzw_decompress(compressedImage, dict)
% 将字节流转换为编码数据流
compressedImage = typecast(compressedImage, 'uint16');
% 初始化解码参数
code = 257;
p = uint8([]);
data = [];
% LZW 解码
for i = 1:numel(compressedImage)
k = compressedImage(i);
if k <= 256
c = dict{k};
else
c = [p dict{k}(1)];
end
data = [data c];
if ~isempty(p)
dict{code} = [p c(1)];
code = code + 1;
end
p = c;
end
% 将一维数据流转换为图像
image = reshape(data, size(image));
end
```
这个函数将输入的压缩后的字节流 `compressedImage` 转换为编码数据流,然后初始化解码参数。接下来,我们对编码数据流进行 LZW 解码,将解码后的数据转换为图像并返回。函数中的解码过程与编码过程不同,因为我们需要在解码过程中构建编码字典。因此,我们使用了一个变量 `code` 来追踪下一个字典条目的编码值。
这是一个简单的 LZW 图像压缩编码实现,可以用于对图像进行无损压缩。需要注意的是,该实现仅支持 8 位灰度图像。如果要支持彩色图像,需要对每个颜色通道分别进行压缩编码。
lzw图像压缩后效果图
LZW图像压缩是一种常用的图像压缩算法。它通过对图像中的重复模式进行编码来减小图像文件的大小。
LZW图像压缩后,效果图会变得更加紧凑。压缩之后,图像文件的大小会显著减小,同时保持大部分的图像质量。在保持相对较高的图像清晰度和细节的同时,LZW压缩还可以有效地减小图像文件的大小,节省磁盘空间。
LZW图像压缩的效果主要取决于图像中的重复模式。如果图像中存在很多重复的像素或者模式,压缩效果会更好,因为LZW会将这些重复的模式以较短的编码进行表示。但如果图像中重复模式较少,那么压缩效果可能会不太明显,甚至可能导致压缩后文件反而变大。
总体而言,LZW图像压缩是一种相对高效的图像压缩算法,可以帮助在不损失太多图像质量的情况下,减小图像文件的大小。但需要注意的是,压缩后的图像需要经过解压缩才能恢复原始的图像,因此在使用时需要确保有相应的解压缩算法。
相关推荐
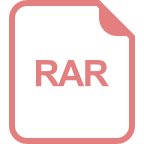












