F.interpolate和F.upsample
时间: 2023-09-07 19:16:51 浏览: 90
`F.interpolate` 和 `F.upsample` 都是PyTorch中用于对图像进行上采样或下采样的函数,不过它们在一些方面是不同的:
1. `F.upsample` 在PyTorch 1.3及以前的版本中使用,而`F.interpolate`是从PyTorch 1.5版本开始推荐使用的。在PyTorch 1.5及以后的版本中, `F.upsample` 已被标记为弃用。
2. `F.interpolate` 可以指定插值方法,如双线性插值、最近邻插值等,而`F.upsample` 只能使用双线性插值。
3. `F.interpolate` 可以指定输出大小,可以是任意的大小,而`F.upsample` 只能指定一个放缩因子。
因此,建议在PyTorch 1.5及以后的版本中使用`F.interpolate`函数,以便更好地控制上下采样的过程。
相关问题
f.interpolate和nn.upsample
### 回答1:
b'f.interpolate' 和 nn.upsample 都是 PyTorch 中用于调整张量大小的函数。具体而言,它们可以用来进行上采样或下采样(即放大或缩小)。其中,b'f.interpolate' 是一个通用的函数,它可以在不同维度上对张量进行自适应的调整大小操作,而 nn.upsample 主要用于对二维图像进行上采样。需要注意的是,由于 b'f.interpolate' 是一个通用的函数,相比之下它的效率可能会稍低一些。
### 回答2:
f.interpolate 和 nn.upsample 是 PyTorch 中常用的两个图像上采样函数,它们的功能都是将一个图像放大到目标大小。但是,它们之间的实现、用法以及适用场景略有不同。
首先,f.interpolate 是一种更加灵活的图像上采样方法,它可以接受一个输入张量和一个目标大小(或缩放因子)作为参数,同时可以选择不同的插值方式来完成上采样操作。其中,插值方式可以是最近邻插值、双线性插值、双三次插值或自定义插值方式。代码如下:
```python
import torch.nn.functional as F
# 缩放因子为2,使用双线性插值
output = F.interpolate(input, scale_factor=2, mode='bilinear', align_corners=False)
# 目标大小为[224, 224],使用最近邻插值
output = F.interpolate(input, size=(224, 224), mode='nearest')
```
nn.upsample 的用法则相对简单,它只需要接受一个输入张量和缩放因子(或目标大小)作为参数,同时不需要指定插值方式。这里需要注意的是,nn.upsample 是一个废弃的函数,推荐使用新的上采样函数 nn.functional.interpolate 代替。代码如下:
```python
import torch.nn as nn
# 缩放因子为2
upsample = nn.Upsample(scale_factor=2)
output = upsample(input)
# 目标大小为[224, 224]
upsample = nn.Upsample(size=(224, 224))
output = upsample(input)
```
从实现上来说,f.interpolate 是通过调用 nn.functional.interpolate 来实现的,因此两者的实现方式是相同的,只是使用方式稍有不同。值得注意的是,f.interpolate 可以适用于几乎所有的上采样场景,而 nn.upsample 已经被废弃,建议不要再使用。
总的来说,如果需要进行图像上采样操作,应该优先考虑使用 f.interpolate,根据实际需求选择不同的插值方式即可。如果仅仅是进行简单的缩放操作,也可以使用 nn.functional.resize 进行操作。
### 回答3:
Pytorch中提供了两种常见的上采样方法:f.interpolate和nn.upsample。这两种方法均可用于对输入的特征图进行上采样,以增加特征图的大小。以下将分别介绍这两种方法的用法和区别。
一、f.interpolate
f.interpolate是一个功能强大的上采样方法,它可以通过设置多个参数来控制上采样的方式,包括上采样的尺寸、上采样的模式、上采样的比例等。f.interpolate支持多种上采样模式,包括:最近邻上采样(nearest)、双线性上采样(bilinear)和三次样条上采样(bicubic)。
使用f.interpolate的方法如下:
```python
import torch.nn.functional as F
out = F.interpolate(x, scale_factor=2, mode='bicubic', align_corners=True)
```
其中,x是输入的特征图,scale_factor设置了上采样的比例,mode设置了上采样的模式(可以选择nearest、bilinear或bicubic),align_corners参数表示是否在计算像素坐标时考虑角点,通常设置为True。
二、nn.upsample
nn.upsample是Pytorch中另一个常用的上采样方法,和f.interpolate相比,它较为简单,只需指定上采样的比例即可。nn.upsample只支持双线性上采样(bilinear),所以不能像f.interpolate一样选择不同的上采样模式。
使用nn.upsample的方法如下:
```python
import torch.nn as nn
upsample = nn.Upsample(scale_factor=2, mode='bilinear', align_corners=True)
out = upsample(x)
```
其中,scale_factor和mode的含义与f.interpolate的一致,也需要设置align_corners参数。
总的来说,f.interpolate的使用更加灵活,支持多种上采样模式,可以通过参数来精细控制上采样的方式。而nn.upsample使用较为简单,只需要指定上采样比例即可。在实际使用中,建议根据需要选择合适的上采样方法。
nn.Upsample
nn.Upsample是PyTorch中的一个上采样操作,用于将输入张量的尺寸放大到指定大小。它可以通过多种插值算法实现,如最近邻插值、双线性插值、三次样条插值等。nn.Upsample的主要参数包括:
- size:指定输出张量的尺寸,可以是int、Tuple[int]或List[int]类型。
- scale_factor:指定输出张量相对于输入张量的缩放比例,可以是float、Tuple[float]或List[float]类型。
- mode:指定插值算法,可以是nearest、linear、bilinear、bicubic或trilinear。
- align_corners:指定是否对齐角点像素。
使用nn.Upsample时,可以直接作为nn.Module的一部分使用,也可以通过nn.functional.interpolate函数调用。例如,以下代码将使用最近邻插值将输入张量大小上采样两倍:
```
import torch.nn as nn
upsample = nn.Upsample(scale_factor=2, mode='nearest')
output = upsample(input_tensor)
```
或者使用函数式调用:
```
import torch.nn.functional as F
output = F.interpolate(input_tensor, scale_factor=2, mode='nearest')
```
相关推荐
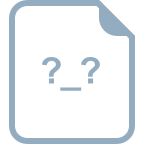











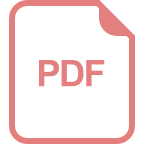