springboot使用LibreOffice来实现文档的转换的示例代码
时间: 2024-02-17 08:00:17 浏览: 39
下面是一个示例代码,演示如何在Spring Boot中使用LibreOffice来实现文档转换:
首先,需要在pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>com.artofsolving</groupId>
<artifactId>jodconverter-core</artifactId>
<version>3.0-beta-4</version>
</dependency>
```
然后,在Java类中注入LibreOffice相关的配置:
```java
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class LibreOfficeConfig {
@Value("${libreoffice.home:}")
private String officeHome;
@Bean
public LibreOfficeConverter libreOfficeConverter() {
return new LibreOfficeConverter(officeHome);
}
}
```
在上述代码中,我们使用了@Value注解来获取配置文件中的"libreoffice.home"属性值,并将其注入到officeHome字段中。然后,我们创建了一个名为"libreOfficeConverter"的Bean,用于将文档转换为PDF格式。
最后,我们可以在Controller中使用此Bean来实现文档转换:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
@RestController
public class DocumentController {
@Autowired
private LibreOfficeConverter libreOfficeConverter;
@PostMapping("/convert")
public String convertDocument(@RequestParam("src") String srcPath,
@RequestParam("dest") String destPath) throws IOException {
File inputFile = new File(srcPath);
File outputFile = new File(destPath);
FileInputStream inputStream = new FileInputStream(inputFile);
FileOutputStream outputStream = new FileOutputStream(outputFile);
libreOfficeConverter.convert(inputStream, outputFile);
inputStream.close();
outputStream.close();
return "Document converted successfully!";
}
}
```
在上述代码中,我们注入了之前创建的"libreOfficeConverter" Bean,并在convertDocument方法中使用它来将文档从输入流转换为PDF格式。最后,我们关闭了输入流和输出流,并返回一个成功转换的提示消息。
需要注意的是,上述代码仅供参考,具体实现可能因环境和需求而异。
相关推荐
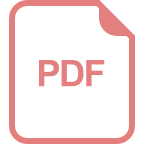
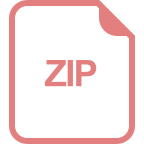
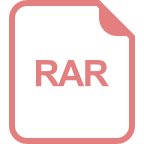














