Linux下,键盘输入文件名,父进程通过管道 发送该文件名给子进程,子进程获取文件名 后判断文件是否存在,之后,将文件是否存 在的信息通过管道告知父进程。
时间: 2024-03-16 13:46:32 浏览: 55
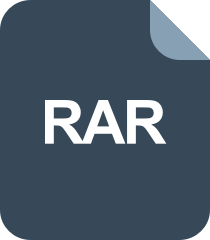
Linux进程间通信之管道
好的,这是一个典型的 Linux 下的进程间通信问题。首先需要创建一个管道,让父进程和子进程都可以读写。然后父进程可以通过读取键盘输入的文件名,并将其通过管道写入到子进程中。子进程可以通过从管道中读取文件名,判断文件是否存在,并将结果通过管道写回给父进程。
下面是对应的 C 语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/wait.h>
#include <sys/types.h>
#include <sys/stat.h>
#define MAX_BUF_SIZE 1024
int main() {
int pipefd[2];
pid_t pid;
char filename[MAX_BUF_SIZE];
char result[MAX_BUF_SIZE];
int file_exists;
/* 创建管道 */
if (pipe(pipefd) == -1) {
perror("pipe");
exit(EXIT_FAILURE);
}
/* 创建子进程 */
pid = fork();
if (pid == -1) {
perror("fork");
exit(EXIT_FAILURE);
}
if (pid == 0) { /* 子进程 */
close(pipefd[1]); /* 关闭写端 */
/* 从管道中读取文件名 */
read(pipefd[0], filename, MAX_BUF_SIZE);
/* 判断文件是否存在 */
if (access(filename, F_OK) == 0) {
file_exists = 1;
} else {
file_exists = 0;
}
/* 将文件是否存在的信息写回给父进程 */
write(pipefd[0], &file_exists, sizeof(file_exists));
close(pipefd[0]);
exit(EXIT_SUCCESS);
} else { /* 父进程 */
close(pipefd[0]); /* 关闭读端 */
/* 读取键盘输入的文件名 */
printf("请输入文件名:");
scanf("%s", filename);
/* 将文件名写入管道 */
write(pipefd[1], filename, MAX_BUF_SIZE);
/* 从管道中读取子进程返回的文件是否存在的信息 */
read(pipefd[1], &file_exists, sizeof(file_exists));
if (file_exists) {
printf("文件存在!\n");
} else {
printf("文件不存在!\n");
}
close(pipefd[1]);
wait(NULL); /* 等待子进程结束 */
exit(EXIT_SUCCESS);
}
}
```
这段代码创建了一个管道 `pipefd`,通过 `fork` 创建子进程,父进程和子进程分别读写管道中的内容。其中,父进程从键盘输入文件名,并将其写入管道中;子进程从管道中读取文件名,判断文件是否存在,并将结果写回给父进程。最后,父进程根据子进程返回的结果输出文件是否存在的信息。
阅读全文
相关推荐
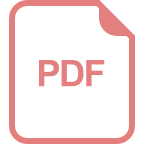
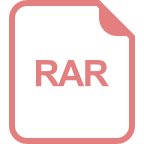
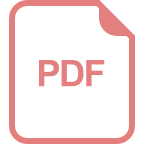
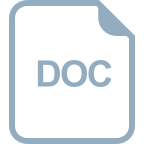
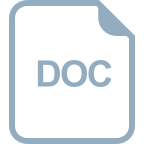
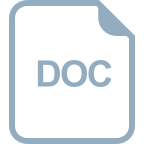
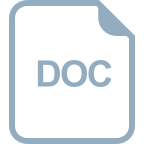
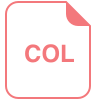
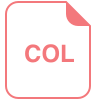
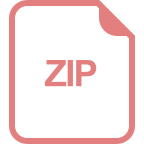
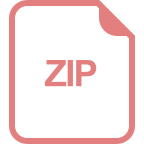
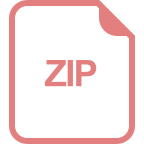