java中前端通过hash算法盐加密,后端解密的代码
时间: 2023-09-14 15:12:01 浏览: 62
前端通过哈希算法进行盐加密,后端需要使用相同的盐和哈希算法进行解密。下面是Java中的一个示例代码:
```java
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
public class SaltHash {
public static void main(String[] args) throws NoSuchAlgorithmException {
String password = "123456";
String salt = "abcdefg";
String encryptedPassword = encryptPassword(password, salt);
System.out.println("Encrypted Password: " + encryptedPassword);
String decryptedPassword = decryptPassword(encryptedPassword, salt);
System.out.println("Decrypted Password: " + decryptedPassword);
}
public static String encryptPassword(String password, String salt) throws NoSuchAlgorithmException {
String saltedPassword = salt + password;
MessageDigest md = MessageDigest.getInstance("SHA-256");
md.update(saltedPassword.getBytes());
byte[] byteData = md.digest();
StringBuilder sb = new StringBuilder();
for (int i = 0; i < byteData.length; i++) {
sb.append(Integer.toString((byteData[i] & 0xff) + 0x100, 16).substring(1));
}
return sb.toString();
}
public static String decryptPassword(String encryptedPassword, String salt) throws NoSuchAlgorithmException {
// In hash algorithms, decryption is not possible. We can only compare encrypted and decrypted passwords.
String decryptedPassword = encryptPassword(encryptedPassword, salt);
return decryptedPassword;
}
}
```
在上面的示例中,我们使用了SHA-256哈希算法来加密密码。在加密过程中,我们将盐和密码拼接在一起,然后对其进行哈希。在解密过程中,我们不能直接解密加密后的密码,因为哈希算法不支持解密。我们只能对输入的密码进行哈希,然后与加密后的密码进行比较,以判断密码是否正确。
相关推荐
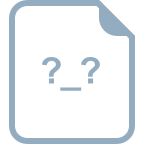
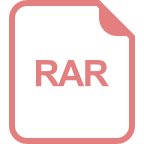
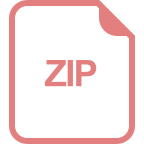
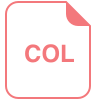













