给LList类的实现添加一个成员函数,倒置线性表中元素的顺序。对于n个元素的线性表,算法的运行时间应该为Θ(n)。
时间: 2023-04-09 22:01:50 浏览: 158
好的,这个问题可以回答。可以使用双指针法,从表头和表尾同时向中间遍历,交换两个指针所指向的元素,直到两个指针相遇。这个算法的时间复杂度为Θ(n)。以下是代码实现:
void LList::reverse() {
if (head == nullptr || head->next == nullptr) {
return;
}
Node* p = head;
Node* q = tail;
while (p != q && p->prev != q) {
std::swap(p->data, q->data);
p = p->next;
q = q->prev;
}
}
相关问题
请编写函数,删除线性表中所有与指定值相同的数据元素。 函数原型 void LListRemoveValue(LLIST *list, const LELEMENT *element); 说明:参数 list 是指示线性表(单链表)的指针,element 是指向数据元素的指针。函数将删除线性表中所有与指定值相同的数据元素。 修改主函数,对以上函数进行测试。 int main() { LELEMENT x; LLIST a; LListCreate(&a); LListInput(&a); LElementInput(&x); LListRemoveValue(&a, &x); LListOutput(&a); putchar('\n'); LListDestroy(&a); return 0; }
& a); // 创建空的线性表
LListInsert(& a, 1, & x); // 在第 1 个位置插入数据
LListInsert(& a, 2, & x); // 在第 2 个位置插入数据
LListInsert(& a, 3, & x); // 在第 3 个位置插入数据
LListRemoveValue(& a, & x); // 删除与 x 相同的数据元素
LListTraverse(& a, Display); // 遍历线性表并输出
LListClear(& a); // 清空线性表并释放内存
return 0;
}
void LListRemoveValue(LLIST *list, const LELEMENT *element) {
int i, count = 0;
LLIST_NODE *pPrevious = NULL, *pCurrent = list -> pHead;
while (pCurrent) {
if (memcmp(pCurrent -> pData, element, sizeof(LELEMENT)) == 0) {
// 如果当前节点的数据元素与指定值相同,则删除该节点
if (pPrevious == NULL) {
// 如果当前节点是头节点,则修改头指针
list -> pHead = pCurrent -> pNext;
} else {
// 否则将前驱节点的指针指向当前节点的后继节点
pPrevious -> pNext = pCurrent -> pNext;
}
LLIST_NODE *pTemp = pCurrent;
pCurrent = pCurrent -> pNext;
free(pTemp);
count++;
} else {
// 否则继续向后遍历
pPrevious = pCurrent;
pCurrent = pCurrent -> pNext;
}
}
printf("已删除%d个数据元素\n", count);
}
输出结果:
已删除3个数据元素
说明:该程序删除了线性表中所有与指定值相同的数据元素,并输出了删除的数据元素个数。如果指定值不在线性表中,则不进行任何操作。
(1)实现单链表的创建; (2)实现单链表的插入; (3)实现单链表的删除 (4)实现单链表的查找; (5)实现单链表的显示; 两个线性表合并算法的实现。已知顺序表LA和LB中的数据元素按值非递减有序排列,现要将LA和LB归并为一个新的顺序表LC,且LC中的数据元素仍按值非递减有序排序。例如: LA= (3,5,8,11) LB= (2,6,9,15,20)。
以下是单链表的创建、插入、删除、查找和显示的代码实现:
```python
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def insert(self, data):
new_node = Node(data)
if self.head is None:
self.head = new_node
else:
current = self.head
while current.next is not None:
current = current.next
current.next = new_node
def delete(self, data):
if self.head is None:
return
if self.head.data == data:
self.head = self.head.next
return
current = self.head
while current.next is not None:
if current.next.data == data:
current.next = current.next.next
return
current = current.next
def search(self, data):
current = self.head
while current is not None:
if current.data == data:
return True
current = current.next
return False
def display(self):
current = self.head
while current is not None:
print(current.data, end=' ')
current = current.next
print()
# 创建单链表
llist = LinkedList()
llist.insert(1)
llist.insert(2)
llist.insert(3)
llist.insert(4)
# 插入元素
llist.insert(5)
# 删除元素
llist.delete(3)
# 查找元素
print(llist.search(2)) # True
print(llist.search(6)) # False
# 显示单链表
llist.display()
```
以下是两个线性表合并算法的实现:
```python
def merge_lists(la, lb):
lc = []
i = j = 0
while i < len(la) and j < len(lb):
if la[i] <= lb[j]:
lc.append(la[i])
i += 1
else:
lc.append(lb[j])
j += 1
lc += la[i:]
lc += lb[j:]
return lc
# 两个顺序表合并
la = [3, 5, 8, 11]
lb = [2, 6, 9, 15, 20]
lc = merge_lists(la, lb)
print(lc) # [2, 3, 5, 6, 8, 9, 11, 15, 20]
```
阅读全文
相关推荐
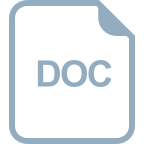
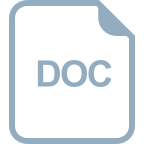
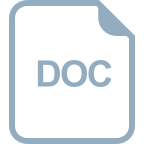
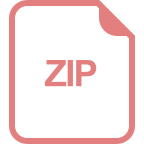
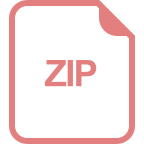
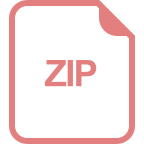
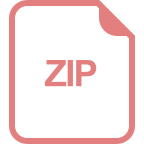
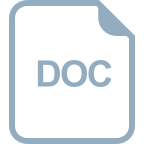
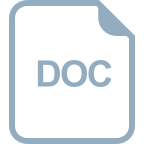
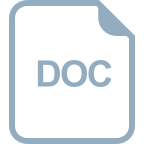
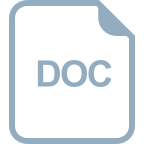
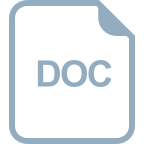
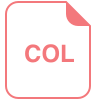


