C++将字符串转为数字的代码
时间: 2023-09-22 08:08:05 浏览: 236
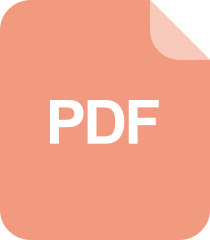
c++将字符串转数字的实例方法
以下是 C++ 中将字符串转化为数字的示例代码:
```
#include <iostream>
#include <string>
int main() {
std::string str_int = "123";
std::string str_float = "3.14";
int num_int = std::stoi(str_int);
float num_float = std::stof(str_float);
std::cout << "The integer value is " << num_int << std::endl;
std::cout << "The float value is " << num_float << std::endl;
return 0;
}
```
以上代码将字符串 `"123"` 转化为整数,字符串 `"3.14"` 转化为浮点数,并输出转化后的结果。需要注意的是,这里使用了 `<string>` 头文件中的 `std::string` 类型。
阅读全文
相关推荐
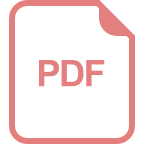



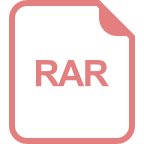
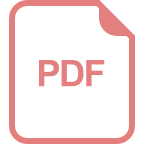










