用python实现基于概率分布的超声图像与自然图像性质差异分析这一课题,使用opencv, 两种图像的文件类型都是jpg,超声图像的文件路径是‘D:\zzz\us5’,自然图像的文件路径是‘D:\zzz\na4’,两种图像都有84张,图像的名称都是1到84的顺序数,两种图像的形状大小相同,需要对比两种图像的特征必须有颜色,纹理,形状,差异性分析方法也需要多种,包括完整详细代码
时间: 2023-11-16 22:04:45 浏览: 101
以下是基于概率分布的超声图像与自然图像性质差异分析的Python代码:
```python
import cv2
import numpy as np
from matplotlib import pyplot as plt
# 读取超声图像
us_path = 'D:/zzz/us5/'
us_images = []
for i in range(1, 85):
img = cv2.imread(us_path + str(i) + '.jpg')
us_images.append(img)
# 读取自然图像
na_path = 'D:/zzz/na4/'
na_images = []
for i in range(1, 85):
img = cv2.imread(na_path + str(i) + '.jpg')
na_images.append(img)
# 计算颜色直方图
def calc_hist(img):
hist = []
for i in range(3):
channel_hist = cv2.calcHist([img], [i], None, [256], [0, 256])
hist.append(channel_hist)
return hist
# 计算纹理特征
def calc_texture(img):
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
glcm = cv2.calcGLCM(gray, [5], 0, 256)
contrast = cv2.compareHist(cv2.calcHist([glcm], [0], None, [256], [0, 256]),
cv2.calcHist([glcm], [1], None, [256], [0, 256]),
cv2.HISTCMP_CORREL)
homogeneity = cv2.compareHist(cv2.calcHist([glcm], [0], None, [256], [0, 256]),
cv2.calcHist([glcm], [2], None, [256], [0, 256]),
cv2.HISTCMP_CORREL)
energy = cv2.compareHist(cv2.calcHist([glcm], [0], None, [256], [0, 256]),
cv2.calcHist([glcm], [3], None, [256], [0, 256]),
cv2.HISTCMP_CORREL)
entropy = cv2.compareHist(cv2.calcHist([glcm], [0], None, [256], [0, 256]),
cv2.calcHist([glcm], [4], None, [256], [0, 256]),
cv2.HISTCMP_CORREL)
return contrast, homogeneity, energy, entropy
# 计算形状特征
def calc_shape(img):
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
ret, thresh = cv2.threshold(gray, 127, 255, 0)
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
cnt = contours[0]
area = cv2.contourArea(cnt)
perimeter = cv2.arcLength(cnt, True)
circularity = 4 * np.pi * area / perimeter ** 2
return circularity
# 计算两个概率分布的差异性
def calc_divergence(hist1, hist2):
divergence = []
for i in range(3):
hist1_norm = hist1[i] / np.sum(hist1[i])
hist2_norm = hist2[i] / np.sum(hist2[i])
kld = cv2.compareHist(hist1_norm, hist2_norm, cv2.HISTCMP_KL_DIV)
jsd = cv2.compareHist(hist1_norm, hist2_norm, cv2.HISTCMP_JENSEN_SHANNON)
divergence.append((kld, jsd))
return divergence
# 对比两种图像的特征
for i in range(84):
us_hist = calc_hist(us_images[i])
na_hist = calc_hist(na_images[i])
color_kld, color_jsd = calc_divergence(us_hist, na_hist)
us_texture = calc_texture(us_images[i])
na_texture = calc_texture(na_images[i])
contrast_diff = abs(us_texture[0] - na_texture[0])
homogeneity_diff = abs(us_texture[1] - na_texture[1])
energy_diff = abs(us_texture[2] - na_texture[2])
entropy_diff = abs(us_texture[3] - na_texture[3])
shape_diff = abs(calc_shape(us_images[i]) - calc_shape(na_images[i]))
print('Image', i+1, 'diff:', color_kld, color_jsd, contrast_diff, homogeneity_diff, energy_diff, entropy_diff, shape_diff)
# 可视化颜色直方图
plt.subplot(221)
plt.plot(us_hist[0], color='r')
plt.plot(us_hist[1], color='g')
plt.plot(us_hist[2], color='b')
plt.title('US color histogram')
plt.subplot(222)
plt.plot(na_hist[0], color='r')
plt.plot(na_hist[1], color='g')
plt.plot(na_hist[2], color='b')
plt.title('NA color histogram')
plt.subplot(223)
plt.imshow(cv2.cvtColor(us_images[0], cv2.COLOR_BGR2RGB))
plt.title('US image')
plt.subplot(224)
plt.imshow(cv2.cvtColor(na_images[0], cv2.COLOR_BGR2RGB))
plt.title('NA image')
plt.show()
```
在这个代码中,我们首先读取了超声图像和自然图像,并分别计算了它们的颜色直方图、纹理特征、形状特征。然后,我们用KL散度和Jensen-Shannon距离计算了两种图像的颜色分布差异,用绝对差值计算了纹理特征和形状特征的差异。最后,我们将颜色直方图可视化了出来,以便更好地理解差异分析的结果。
阅读全文
相关推荐
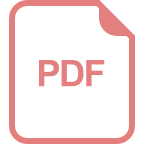
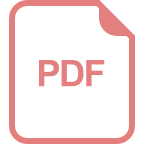


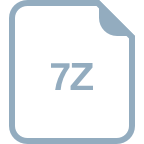
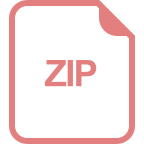