PreparedStatement pstmt = con.prepareStatement(sql); pstmt.setString(1, bookType.getBookTypeName()); pstmt.setString(2, bookType.getBookTypeDesc())这几条语句是什么意思
时间: 2024-04-09 14:27:16 浏览: 89
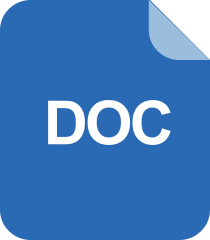
JDBC及java.sql包简介

这几条语句是使用 PreparedStatement 对象来执行 SQL 查询或更新操作的步骤。
1. `PreparedStatement pstmt = con.prepareStatement(sql);`
- 这行代码创建了一个 PreparedStatement 对象 `pstmt`,它被用来执行预编译的 SQL 语句。
- `con` 是一个已经建立的数据库连接对象。
- `sql` 是包含 SQL 查询或更新语句的字符串。
2. `pstmt.setString(1, bookType.getBookTypeName());`
- 这行代码设置了第一个参数的值。
- `setString()` 方法用于设置指定参数的值为字符串类型。
- `1` 是占位符的位置,表示第一个参数。
- `bookType.getBookTypeName()` 返回一个书籍类型的名称,它将被设置为第一个参数的值。
3. `pstmt.setString(2, bookType.getBookTypeDesc());`
- 这行代码设置了第二个参数的值。
- 同样使用 `setString()` 方法,将第二个参数设置为书籍类型的描述。
这些语句的目的是为了将 Java 对象中的属性值设置到预编译的 SQL 语句中的占位符上,以便执行后续的 SQL 查询或更新操作。
阅读全文
相关推荐
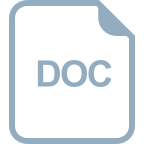
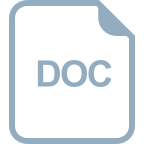
















