编写程序,把24小时表示法的时间转换为12小时表示法。定义名为TimeFormatException的异常类,如果输入的时间是非法值,比如10:65或者abc,程序应该抛出并处理TimeFormatException异常。 要求编程实现TimeFormatException类,并实现Test类中的outTime()方法,使给定的Test类能正常运行,并实现指定的输出内容。 提示:时间格式转换可以使用java.text.SimpleDateFormat类,具体用法自行查看API文档或网上资料。 public class Test{ public static void main(String[] args) { String times[] = {"15:20","27:10","16:78","abc","6:30"}; String line = null; for (String timeStr:times ) { System.out.println("==="); System.out.println("<<<TimeString for format converting in 24-hour notation is "+ timeStr); try { outTime(timeStr); } catch (TimeFormatException e) { System.out.println(e); continue; } } System.out.println("End of program"); } public static void outTime(String line) throws TimeFormatException { } }
时间: 2023-06-18 21:07:52 浏览: 89
可以按照以下方式实现Test类中的outTime()方法:
```
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
public class Test {
public static void main(String[] args) {
String times[] = {"15:20", "27:10", "16:78", "abc", "6:30"};
String line = null;
for (String timeStr : times) {
System.out.println("===");
System.out.println("<<<TimeString for format converting in 24-hour notation is " + timeStr);
try {
outTime(timeStr);
} catch (TimeFormatException e) {
System.out.println(e);
continue;
}
}
System.out.println("End of program");
}
public static void outTime(String line) throws TimeFormatException {
SimpleDateFormat inputFormat = new SimpleDateFormat("HH:mm");
SimpleDateFormat outputFormat = new SimpleDateFormat("hh:mm a");
try {
Date date = inputFormat.parse(line);
String output = outputFormat.format(date);
System.out.println(">>>TimeString for format converting in 12-hour notation is " + output);
} catch (ParseException e) {
throw new TimeFormatException("Invalid time format: " + line);
}
}
}
class TimeFormatException extends Exception {
public TimeFormatException(String message) {
super(message);
}
}
```
在outTime()方法中,首先创建了两个SimpleDateFormat对象,一个用于解析输入的时间字符串,另一个用于格式化输出的时间字符串。然后使用inputFormat对象将输入的时间字符串解析为一个Date对象,再使用outputFormat对象将Date对象格式化为12小时制的时间字符串。如果输入的时间字符串不符合规范,就抛出TimeFormatException异常。在main()方法中,如果outTime()方法抛出了TimeFormatException异常,就捕获并输出异常信息,然后继续处理下一个时间字符串。最后输出程序结束的提示信息。
阅读全文
相关推荐
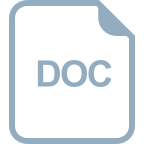
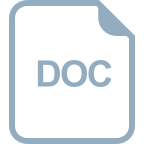
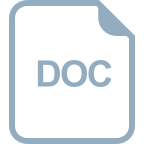
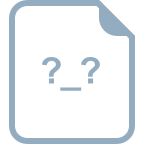
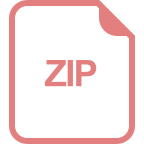
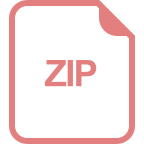
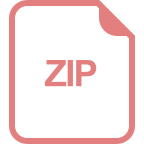
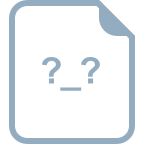
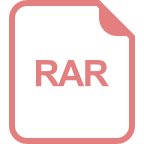
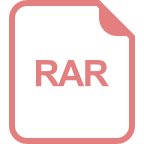
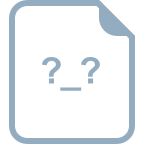
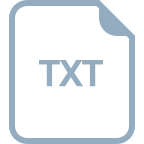
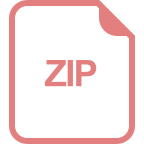
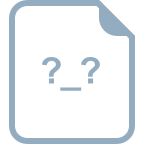
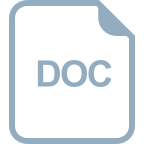