写一个基于onnxruntime的onnx模型,实时检测的python代码
时间: 2023-09-17 21:06:10 浏览: 84
### 回答1:
您好,您的问题是如何编写基于onnxruntime的onnx模型的实时检测Python代码。以下是一个简单的示例,用于加载onnx模型并对输入数据进行即时检测。
```
import onnxruntime as ort
import numpy as np
# 加载模型
model = ort.InferenceSession('model.onnx')
# 设置输入和输出名称
input_name = model.get_inputs()[0].name
output_name = model.get_outputs()[0].name
# 创建模拟输入数据
input_data = np.random.rand(1, 3, 224, 224).astype(np.float32)
# 进行预测
output = model.run([output_name], {input_name: input_data})
# 输出检测结果
print(output)
```
请注意,此示例假定您已经拥有了一个训练好的onnx模型,并已将其保存为 `model.onnx` 文件。在实际情况中,您需要根据您自己的数据和模型进行相应的调整。
希望这个示例能为您提供有用的指导,如果您有任何其他问题,请随时提出。
### 回答2:
import cv2
import numpy as np
import onnxruntime as ort
# 加载onnx模型
model_path = 'model.onnx'
session = ort.InferenceSession(model_path)
# 设置输入输出名称
input_name = session.get_inputs()[0].name
output_name = session.get_outputs()[0].name
# 加载分类标签
class_labels = ['label1', 'label2', 'label3', ...]
# 实时检测
cap = cv2.VideoCapture(0)
while True:
ret, frame = cap.read()
# 预处理图像
input_data = cv2.resize(frame, (224, 224))
input_data = np.expand_dims(input_data, axis=0)
input_data = input_data.astype(np.float32) / 255.0
input_data = np.transpose(input_data, (0, 3, 1, 2)) # 调整通道顺序
# 运行推理
outputs = session.run([output_name], {input_name: input_data})
# 解析输出
output_data = outputs[0][0]
predicted_class_index = np.argmax(output_data)
predicted_class_label = class_labels[predicted_class_index]
# 在图像上标注结果
cv2.putText(frame, predicted_class_label, (10, 30),
cv2.FONT_HERSHEY_SIMPLEX, 1, (0, 255, 0), 2)
# 显示图像
cv2.imshow('Real-time Detection', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# 释放资源
cap.release()
cv2.destroyAllWindows()
### 回答3:
基于onnxruntime的onnx模型实时检测的Python代码可以如下:
首先,需要安装onnxruntime库和其他必要的依赖包:
```python
pip install onnxruntime
```
然后,加载并初始化模型:
```python
import onnxruntime as ort
import numpy as np
# 加载模型
model_path = 'path_to_your_model.onnx'
session = ort.InferenceSession(model_path)
# 获取输入和输出名称
input_name = session.get_inputs()[0].name
output_name = session.get_outputs()[0].name
```
接下来,准备输入数据并进行模型推理:
```python
def detect(image):
# 图像预处理,根据模型要求进行尺寸调整、归一化等操作
resized_image = preprocess_image(image)
# 图像转为numpy数组并增加batch维度
input_data = np.expand_dims(resized_image, axis=0)
# 进行模型推理
output = session.run([output_name], {input_name: input_data})
# 处理输出结果并返回检测结果
detection_result = post_process_output(output)
return detection_result
```
在上述代码中,`preprocess_image`函数用于对图像进行预处理,例如调整尺寸、归一化等操作。`post_process_output`函数用于处理模型输出,例如解码检测框、进行类别分类等操作。
最后,在主程序中调用`detect`函数进行实时检测,例如:
```python
import cv2
# 初始化摄像头
camera = cv2.VideoCapture(0)
while True:
# 读取图像帧
ret, frame = camera.read()
# 进行实时检测
result = detect(frame)
# 在图像上绘制检测框等信息
frame_with_result = draw_detection_result(frame, result)
# 显示图像帧
cv2.imshow('Real-time Detection', frame_with_result)
# 按下ESC键退出
if cv2.waitKey(1) == 27:
break
# 释放资源
camera.release()
cv2.destroyAllWindows()
```
上述代码中,`draw_detection_result`函数用于在图像上绘制检测结果,例如绘制检测框、标注类别等信息。在主循环中,不断从摄像头读取图像帧并进行实时检测,并在图像上显示检测结果。
请注意,以上代码仅为示例,实际应用中需要根据具体任务和模型的需求进行适当的修改和优化。
相关推荐
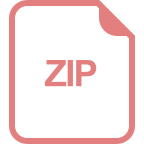
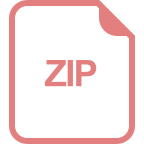
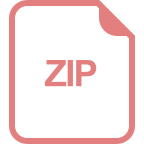














