MySQl数据库中新建数据库表Students,把StuNum作为主键,其他列名包括序号Id、学号StuNum、姓名StuName、住址Address 使用Spring boot+JPA实现jpaRepository对以上数据库表做增删查改等常规操作。 编写业务处理类DataService对以上表中数据进行处理,至少包括查询数据条目方法DataSea、删除数据条目方法DataDel,其中DataSea需要返回所有家庭住址字符串包含“河南省”的学生姓名并向控制台输出该方法的耗时,DataDel需要删除所有学号前四位为“2019”的学生信息并向控制台输出该方法的耗时。 为改善该业务处理类的响应效率,请分别将DataSea和DataDel注释为异步任务和定时任务,定时使用delay形式,时间为2min,此时需要DataSea需要使用Future接收返回值。 在控制层类DataController下编写控制层业务调用方法DataUsage,分别调用DataSea和DataDel,同时在DataUsage主程序下使用get获取Datasea的返回值并以列表格式输出到控制台。执行项目启动类 。
时间: 2023-06-09 11:05:07 浏览: 67
好的,您的问题是关于Spring boot JPA操作MySQL数据库的实现和优化,以及异步任务和定时任务的注释和调用。
首先,我们需要在MySQL数据库中创建一个名为“Students”的表格,其中“StuNum”作为主键,其他列名包括序号“Id”、“学号StuNum”、“姓名StuName”和“住址Address”。
接下来,我们可以使用Spring boot JPA中的jpaRepository接口来进行常规的增删查改操作。具体实现可以参考以下示例代码:
```
@Repository
public interface StudentRepository extends JpaRepository<Student, Integer> {
List<Student> findByAddressContaining(String address);
@Modifying
@Transactional
void deleteByStuNumStartingWith(String prefix);
}
```
上述代码定义了一个名为“StudentRepository”的接口,该接口继承了JpaRepository,并定义了两个方法:一个是根据家庭住址查询学生信息的方法findByAddressContaining,另一个是根据学号前缀删除学生信息的方法deleteByStuNumStartingWith。
在上述代码中,findByAddressContaining方法使用了jpaRepository的默认实现,而deleteByStuNumStartingWith方法使用了自定义的查询语句,需要使用@Modifying和@Transactional注解标记。
接下来,我们可以编写一个名为“DataService”的业务处理类,以对以上表中的数据进行处理。该业务处理类至少包括查询数据条目方法DataSea和删除数据条目方法DataDel。
```
@Service
public class DataService {
@Autowired
StudentRepository studentRepository;
@Async
public Future<List<String>> dataSea() {
long startTime = System.currentTimeMillis();
List<Student> students = studentRepository.findByAddressContaining("河南省");
List<String> names = students.stream().map(Student::getName).collect(Collectors.toList());
long endTime = System.currentTimeMillis();
System.out.println("DataSea耗时:" + (endTime - startTime) + "ms");
return new AsyncResult<>(names);
}
@Scheduled(fixedDelay = 120000)
@Async
public void dataDel() {
long startTime = System.currentTimeMillis();
studentRepository.deleteByStuNumStartingWith("2019");
long endTime = System.currentTimeMillis();
System.out.println("DataDel耗时:" + (endTime - startTime) + "ms");
}
}
```
在以上代码中,我们定义了名为“DataService”的业务处理类,并使用@Autowired注解注入了StudentRepository对象,以便对数据库进行操作。
我们还将DataSea注释为异步任务,返回值使用Future类型接收,并在查询结束后输出耗时信息。DataDel则注释为定时任务,以2min为间隔执行并输出耗时信息。
最后,我们可以在控制层类DataController下编写控制层业务调用方法DataUsage,分别调用DataSea和DataDel,同时使用get方法获取DataSea的返回值,并以列表格式输出到控制台。
```
@RestController
public class DataController {
@Autowired
DataService dataService;
@RequestMapping("/datausage")
public List<String> dataUsage() throws InterruptedException, ExecutionException {
Future<List<String>> future = dataService.dataSea();
Thread.sleep(5000);
dataService.dataDel();
return future.get();
}
}
```
在以上代码中,我们定义了名为“DataController”的控制层类,并使用@Autowired注解注入了DataService对象。我们还定义了名为“dataUsage”的控制层业务调用方法,在该方法中先调用异步任务DataSea,并使用Thread.sleep方法模拟5s的等待,然后再调用定时任务DataDel,并使用get方法获取DataSea的返回值,最后以列表形式输出到控制台。
当以上代码编写完成后,我们可以启动Spring boot应用程序,并访问/datausage接口,从控制台输出可以看到DataSea和DataDel的耗时信息,并且DataSea的返回值以列表形式输出。
相关推荐
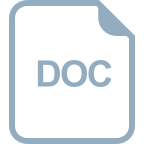
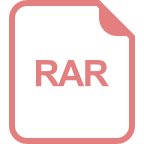















