在Controller层写一个树形结构json
时间: 2024-05-13 12:15:38 浏览: 53
假设你有一个树形结构的数据,如下:
```
[
{
"id": 1,
"name": "Parent 1",
"children": [
{
"id": 2,
"name": "Child 1 of Parent 1",
"children": [
{
"id": 4,
"name": "Grandchild 1 of Child 1 of Parent 1",
"children": []
},
{
"id": 5,
"name": "Grandchild 2 of Child 1 of Parent 1",
"children": []
}
]
},
{
"id": 3,
"name": "Child 2 of Parent 1",
"children": []
}
]
},
{
"id": 6,
"name": "Parent 2",
"children": [
{
"id": 7,
"name": "Child 1 of Parent 2",
"children": [
{
"id": 8,
"name": "Grandchild 1 of Child 1 of Parent 2",
"children": []
}
]
}
]
}
]
```
在 Controller 层中,你可以使用 Laravel 的 Response 类来返回一个 JSON 格式的数据。你需要将树形结构的数据转换为 JSON 格式的字符串,然后使用 Response 类将其返回。
你可以使用 PHP 的内置函数 json_encode 将数据转换为 JSON 格式的字符串。然后,你可以使用 Response 类的 json 方法将 JSON 格式的字符串作为响应返回。如下所示:
```php
public function tree()
{
$data = [
[
"id" => 1,
"name" => "Parent 1",
"children" => [
[
"id" => 2,
"name" => "Child 1 of Parent 1",
"children" => [
[
"id" => 4,
"name" => "Grandchild 1 of Child 1 of Parent 1",
"children" => []
],
[
"id" => 5,
"name" => "Grandchild 2 of Child 1 of Parent 1",
"children" => []
]
]
],
[
"id" => 3,
"name" => "Child 2 of Parent 1",
"children" => []
]
]
],
[
"id" => 6,
"name" => "Parent 2",
"children" => [
[
"id" => 7,
"name" => "Child 1 of Parent 2",
"children" => [
[
"id" => 8,
"name" => "Grandchild 1 of Child 1 of Parent 2",
"children" => []
]
]
]
]
]
];
return response()->json($data);
}
```
在上面的例子中,我们将树形结构的数据保存在 $data 变量中。然后,我们使用 Laravel 的 Response 类将数据转换为 JSON 格式的字符串,并将其作为响应返回。
阅读全文
相关推荐
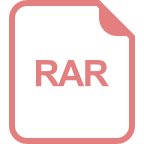
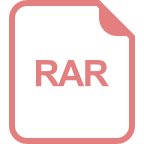


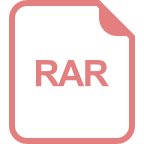




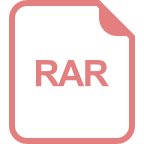


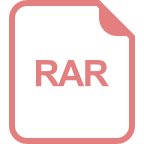


