用pytorch写一个黎曼卷积层orch
时间: 2024-05-04 19:18:27 浏览: 158
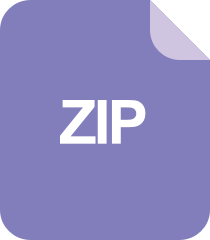
python_卷积层的[1412.6553]和[1511.06530]张量分解方法的Pytorch实现
黎曼卷积层是一种基于黎曼流形的卷积操作,可以用来处理在流形上定义的数据,比如图像、声音等。下面是一个基于PyTorch实现黎曼卷积层的示例代码。
首先,我们需要导入PyTorch和其他相关的库:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
from collections import OrderedDict
```
接下来,我们定义黎曼卷积层的类:
```python
class RiemannConv(nn.Module):
def __init__(self, in_channels, out_channels, kernel_size, manifold, stride=1, padding=0, dilation=1, groups=1, bias=True):
super(RiemannConv, self).__init__()
self.in_channels = in_channels
self.out_channels = out_channels
self.kernel_size = kernel_size
self.stride = stride
self.padding = padding
self.dilation = dilation
self.groups = groups
# 首先定义一个普通的卷积层
self.conv = nn.Conv2d(in_channels, out_channels, kernel_size, stride=stride, padding=padding, dilation=dilation, groups=groups, bias=bias)
# 然后初始化黎曼流形相关的参数
self.manifold = manifold
self.manifold_dim = manifold.dim()
self.weight = nn.Parameter(torch.Tensor(out_channels, in_channels // groups, kernel_size[0], kernel_size[1], self.manifold_dim, self.manifold_dim))
if bias:
self.bias = nn.Parameter(torch.Tensor(out_channels))
else:
self.register_parameter('bias', None)
self.reset_parameters()
```
在这个类中,我们首先定义了一些基本的卷积参数,例如输入和输出通道数、卷积核大小等。然后我们定义了一个普通的`nn.Conv2d`卷积层,用于处理普通的欧几里得数据。
接下来,我们定义了一些与黎曼流形有关的参数,例如流形的维度、权重矩阵的形状等。我们还定义了一个权重矩阵`self.weight`和一个偏置向量`self.bias`,它们都是可训练的参数。
最后,我们调用了`reset_parameters`函数,用于初始化模型参数:
```python
def reset_parameters(self):
stdv = 1. / (self.weight.size(-1) * self.weight.size(-2))
self.weight.data.uniform_(-stdv, stdv)
if self.bias is not None:
self.bias.data.zero_()
```
这个函数用于将权重矩阵和偏置向量初始化为随机值。
接下来,我们需要实现黎曼卷积的前向传播函数:
```python
def forward(self, input):
# 获取输入数据的形状
batch_size, in_channels, height, width = input.size()
# 将输入数据转换成流形张量
input_manifold = self.manifold.from_vector(input.view(batch_size, in_channels, height * width))
# 将权重矩阵转换成流形张量
weight_manifold = self.manifold.from_vector(self.weight.view(self.out_channels, self.in_channels // self.groups, self.kernel_size[0] * self.kernel_size[1] * self.manifold_dim * self.manifold_dim))
# 计算卷积
output_manifold = self.manifold.convolution(weight_manifold, input_manifold, stride=self.stride, padding=self.padding, dilation=self.dilation, groups=self.groups)
# 将输出流形张量转换成普通张量
output = output_manifold.to_vector().view(batch_size, self.out_channels, height, width)
# 添加偏置项
if self.bias is not None:
output += self.bias.view(1, self.out_channels, 1, 1).expand_as(output)
return output
```
这个函数首先将输入数据转换成流形张量,然后将权重矩阵也转换成流形张量。接着,我们调用黎曼流形的卷积函数`self.manifold.convolution`,计算卷积结果。最后,我们将输出流形张量转换成普通张量,添加偏置项,并返回结果。
最后,我们可以使用上面定义的`RiemannConv`类来构建一个神经网络,例如:
```python
class MyNet(nn.Module):
def __init__(self, manifold):
super(MyNet, self).__init__()
self.conv1 = RiemannConv(3, 16, kernel_size=3, manifold=manifold)
self.conv2 = RiemannConv(16, 32, kernel_size=3, manifold=manifold)
self.fc1 = nn.Linear(32 * 7 * 7, 128)
self.fc2 = nn.Linear(128, 10)
def forward(self, x):
x = F.relu(self.conv1(x))
x = F.relu(self.conv2(x))
x = x.view(x.size(0), -1)
x = F.relu(self.fc1(x))
x = self.fc2(x)
return x
```
在这个网络中,我们使用了两个黎曼卷积层和两个全连接层。我们可以使用这个网络来处理在黎曼流形上定义的数据,例如球面图像或声音信号。
阅读全文
相关推荐
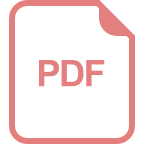
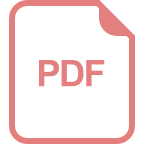
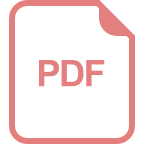
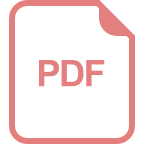
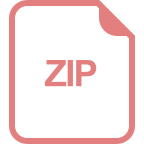
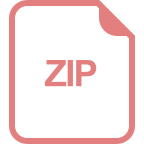
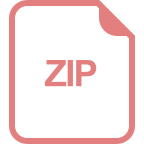
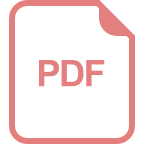







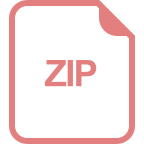