用C++写一个定时器线程,每小时触发一次
时间: 2024-03-08 22:46:54 浏览: 72
可以使用C++11中提供的std::thread和std::chrono库来实现定时器线程。具体实现方法如下:
```cpp
#include <iostream>
#include <thread>
#include <chrono>
using namespace std;
void timer_thread()
{
while (true)
{
// 等待1小时
this_thread::sleep_for(chrono::hours(1));
// 触发定时器事件
cout << "Timer event triggered." << endl;
}
}
int main()
{
// 创建定时器线程
thread t(timer_thread);
// 等待线程结束
t.join();
return 0;
}
```
上述代码中,timer_thread()函数是定时器线程的函数体,其中使用了this_thread::sleep_for()函数来等待1小时,然后触发定时器事件。在main()函数中,创建了定时器线程t,并等待线程结束。
相关问题
用C++写一个定时器线程,每小时触发一次,创建时间文件夹,文件夹名称年月日时
可以在上面的定时器线程的基础上,加入创建时间文件夹的代码。具体实现方法如下:
```cpp
#include <iostream>
#include <thread>
#include <chrono>
#include <ctime>
#include <string>
#include <sys/stat.h>
using namespace std;
// 获取当前时间,返回格式为年月日时的字符串
string get_current_time()
{
time_t t = time(nullptr);
struct tm *now = localtime(&t);
char buf[80];
strftime(buf, sizeof(buf), "%Y%m%d%H", now);
return buf;
}
void timer_thread()
{
while (true)
{
// 等待1小时
this_thread::sleep_for(chrono::hours(1));
// 获取当前时间
string time_str = get_current_time();
// 创建时间文件夹
string folder_name = time_str + "/";
mkdir(folder_name.c_str(), 0777);
// 输出日志
cout << "Folder " << folder_name << " created." << endl;
}
}
int main()
{
// 创建定时器线程
thread t(timer_thread);
// 等待线程结束
t.join();
return 0;
}
```
上述代码中,get_current_time()函数用于获取当前时间,并返回格式为年月日时的字符串。在timer_thread()函数中,先等待1小时,然后获取当前时间,创建时间文件夹,并输出日志。在main()函数中,创建了定时器线程t,并等待线程结束。
ubuntu c++ 写个10秒的定时器
当你提到 "10秒的定时器",我理解为你希望在程序中设置一个定时器,每隔10秒触发一次某个操作。以下是一个示例代码:
```cpp
#include <iostream>
#include <chrono>
#include <thread>
void timerCallback() {
std::cout << "定时器触发!" << std::endl;
// 这里可以执行你想要的操作
}
int main() {
std::cout << "启动定时器..." << std::endl;
while (true) {
// 等待 10 秒钟
std::this_thread::sleep_for(std::chrono::seconds(10));
// 定时器触发,执行回调函数
timerCallback();
}
return 0;
}
```
这段代码使用了 `<iostream>` 来进行输入输出操作,`<chrono>` 来进行时间相关的操作,`<thread>` 来进行线程相关的操作。我们定义了一个名为 `timerCallback` 的回调函数,用于在定时器触发时执行某个操作。在 `main` 函数中,我们首先输出 "启动定时器...",然后进入一个无限循环。在每次循环中,我们使用 `std::this_thread::sleep_for` 函数来让程序暂停 10 秒钟,然后调用 `timerCallback` 函数来执行定时器操作。
你可以将以上代码复制到一个文件(例如 `timer.cpp`),然后使用以下命令在 Ubuntu 上编译和运行它:
```
g++ timer.cpp -o timer
./timer
```
这将编译代码并生成一个名为 `timer` 的可执行文件。运行 `./timer` 命令后,你将会看到 "启动定时器...",然后程序会每隔 10 秒钟输出 "定时器触发!"。你可以根据需要在 `timerCallback` 函数中编写其他操作。如果需要终止定时器,你可以在循环中添加相应的条件来跳出循环。
阅读全文
相关推荐
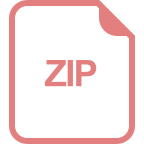
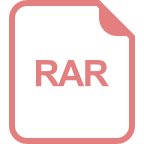
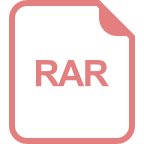
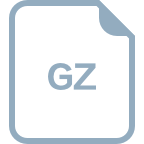
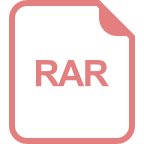
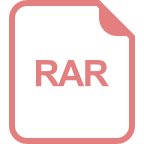
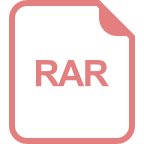
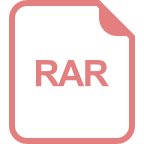
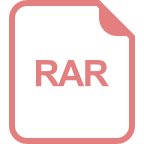
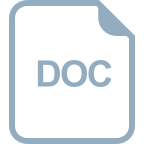
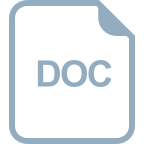
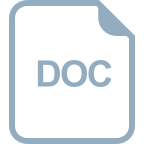
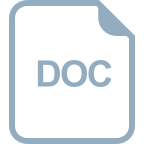
