通过javaScript实现Vue的双向数据绑定
时间: 2024-05-09 10:15:49 浏览: 6
Vue的双向数据绑定是通过使用数据劫持和发布-订阅模式来实现的。以下是一个简单的实现:
首先,我们需要创建一个观察者对象,它将负责收集依赖项和通知订阅者更新:
```javascript
class Watcher {
constructor(vm, expOrFn, cb) {
this.vm = vm;
this.getter = parsePath(expOrFn);
this.cb = cb;
this.value = this.get();
}
get() {
Dep.target = this;
const value = this.getter.call(this.vm, this.vm);
Dep.target = null;
return value;
}
update() {
const oldValue = this.value;
this.value = this.get();
this.cb.call(this.vm, this.value, oldValue);
}
}
```
接下来,我们需要创建一个依赖项对象,它将负责管理依赖项和通知观察者更新:
```javascript
class Dep {
constructor() {
this.subs = [];
}
addSub(sub) {
this.subs.push(sub);
}
removeSub(sub) {
remove(this.subs, sub);
}
depend() {
if (Dep.target) {
Dep.target.addDep(this);
}
}
notify() {
const subs = this.subs.slice();
for (let i = 0, l = subs.length; i < l; i++) {
subs[i].update();
}
}
}
Dep.target = null;
```
最后,我们需要创建一个代理对象,它将负责将实际的数据对象和观察者对象联系起来:
```javascript
function observe(value) {
if (!value || typeof value !== 'object') {
return;
}
Object.keys(value).forEach(key => {
defineReactive(value, key, value[key]);
});
}
function defineReactive(obj, key, val) {
const dep = new Dep();
Object.defineProperty(obj, key, {
enumerable: true,
configurable: true,
get() {
dep.depend();
return val;
},
set(newVal) {
if (val === newVal) {
return;
}
val = newVal;
dep.notify();
},
});
}
function proxy(target, sourceKey) {
Object.keys(target[sourceKey]).forEach(key => {
Object.defineProperty(target, key, {
enumerable: true,
configurable: true,
get() {
return target[sourceKey][key];
},
set(newVal) {
target[sourceKey][key] = newVal;
},
});
});
}
class Vue {
constructor(options) {
this.$options = options;
this._data = options.data;
observe(this._data);
proxy(this, '_data');
}
}
```
这就是一个简单的Vue双向数据绑定的实现。当数据发生变化时,观察者对象将会被通知,并且视图将会自动更新。
相关推荐
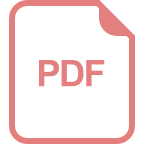
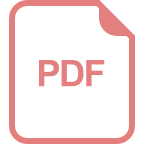
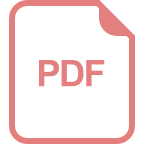














