java里SQL insert操作的语法_SQL基础语法的单表操作 select|insert|update|delete(增删改查) 简单使用...
时间: 2023-12-06 13:05:11 浏览: 130
在Java中进行SQL的insert操作,需要执行以下步骤:
1. 加载JDBC驱动程序
```java
Class.forName("com.mysql.jdbc.Driver"); // 加载MySQL JDBC驱动程序
```
2. 创建数据库连接
```java
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/test", "root", "password");
```
其中,`jdbc:mysql://localhost:3306/test`表示连接的是本地的MySQL数据库,端口号为3306,数据库名为test。`root`和`password`分别是MySQL数据库的用户名和密码。
3. 创建Statement对象
```java
Statement stmt = conn.createStatement();
```
4. 执行SQL语句
```java
String sql = "INSERT INTO user(id, name, age) VALUES(1, '张三', 20)";
stmt.executeUpdate(sql);
```
其中,`sql`为要执行的SQL语句,`executeUpdate()`方法用于执行insert、update、delete等操作。
5. 关闭Statement和连接
```java
stmt.close();
conn.close();
```
完整代码如下:
```java
import java.sql.*;
public class InsertDemo {
public static void main(String[] args) {
try {
// 加载MySQL JDBC驱动程序
Class.forName("com.mysql.jdbc.Driver");
// 创建数据库连接
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/test", "root", "password");
// 创建Statement对象
Statement stmt = conn.createStatement();
// 执行SQL语句
String sql = "INSERT INTO user(id, name, age) VALUES(1, '张三', 20)";
stmt.executeUpdate(sql);
// 关闭Statement和连接
stmt.close();
conn.close();
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
```
在实际开发中,为了避免SQL注入等安全问题,建议使用PreparedStatement来执行SQL语句。PreparedStatement使用起来与Statement类似,只是需要将SQL语句中的参数使用占位符(?)代替,然后在执行SQL语句前设置参数的值即可。
阅读全文
相关推荐
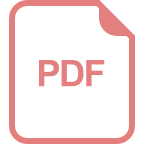
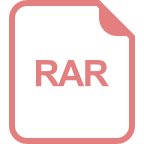
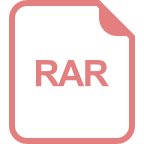
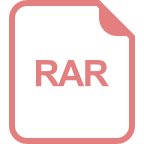
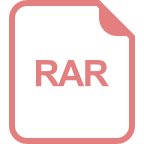
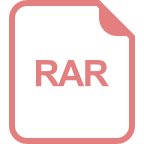
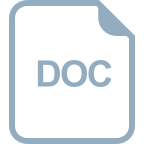
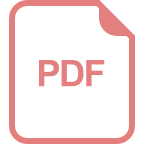
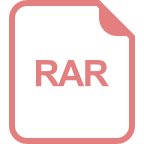
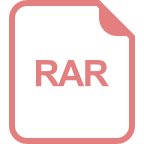
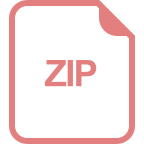
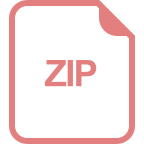
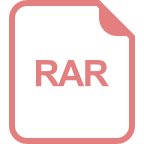
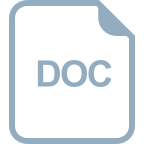



