生成一个简单的餐厅管理系统的c语言代码
时间: 2023-08-04 21:05:07 浏览: 49
好的,以下是一个简单的餐厅管理系统的C语言代码示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_MENU_ITEMS 100
#define MAX_ORDER_ITEMS 100
// 菜单项结构体
typedef struct {
char name[50];
float price;
} MenuItem;
// 订单项结构体
typedef struct {
MenuItem item;
int quantity;
} OrderItem;
// 全局变量
MenuItem menu[MAX_MENU_ITEMS];
OrderItem order[MAX_ORDER_ITEMS];
int menuSize = 0;
int orderSize = 0;
// 添加菜单项
void addMenuItem(char name[], float price) {
MenuItem item;
strcpy(item.name, name);
item.price = price;
menu[menuSize++] = item;
}
// 显示菜单
void showMenu() {
printf("Menu:\n");
for (int i = 0; i < menuSize; i++) {
printf("%d. %s - $%.2f\n", i + 1, menu[i].name, menu[i].price);
}
}
// 添加订单项
void addOrderItem(int index, int quantity) {
OrderItem item;
item.item = menu[index - 1];
item.quantity = quantity;
order[orderSize++] = item;
}
// 显示订单
void showOrder() {
printf("Order:\n");
float total = 0;
for (int i = 0; i < orderSize; i++) {
OrderItem item = order[i];
float subtotal = item.item.price * item.quantity;
printf("%d. %s - $%.2f x %d = $%.2f\n", i + 1, item.item.name, item.item.price, item.quantity, subtotal);
total += subtotal;
}
printf("Total: $%.2f\n", total);
}
// 主函数
int main() {
// 添加菜单项
addMenuItem("Hamburger", 5.99);
addMenuItem("Cheeseburger", 6.99);
addMenuItem("French Fries", 2.99);
addMenuItem("Onion Rings", 3.99);
// 显示菜单
showMenu();
// 获取用户输入
int choice, quantity;
printf("Enter choice (0 to exit): ");
scanf("%d", &choice);
// 处理用户输入
while (choice != 0) {
printf("Enter quantity: ");
scanf("%d", &quantity);
addOrderItem(choice, quantity);
showOrder();
printf("Enter choice (0 to exit): ");
scanf("%d", &choice);
}
return 0;
}
```
这个代码实现了一个简单的餐厅管理系统,用户可以查看菜单、选择菜品和数量,系统会自动计算订单总价并显示。当用户输入 0 时,程序退出。这只是一个简单的示例,实际的餐厅管理系统需要更复杂的功能和更完善的界面。
相关推荐
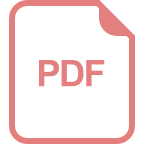
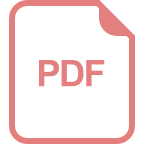
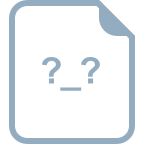















