仿照windows记事本,制作一个记事本软件,实现纯文本文件的新建、打开、保存、另存、复制、粘贴、查找、替换、关于等等功能;关闭软件是弹出对话框提示是否把修改保存,根据用户选择更新磁盘文件内容 java直接给出代码
时间: 2023-06-24 18:04:37 浏览: 48
以下是一个简单的Java记事本程序,实现了新建、打开、保存、另存、复制、粘贴、查找、替换、关于等功能:
```java
import java.awt.BorderLayout;
import java.awt.Container;
import java.awt.Dimension;
import java.awt.FlowLayout;
import java.awt.Font;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import java.util.Scanner;
import javax.swing.Box;
import javax.swing.JButton;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JMenu;
import javax.swing.JMenuBar;
import javax.swing.JMenuItem;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
import javax.swing.KeyStroke;
import javax.swing.WindowConstants;
public class Notepad extends JFrame implements ActionListener, KeyListener {
private static final long serialVersionUID = 1L;
private JTextArea textArea;
private JLabel statusLabel;
private File currentFile;
private boolean modified = false;
public Notepad() {
super("记事本");
Container contentPane = getContentPane();
textArea = new JTextArea();
textArea.setFont(new Font("宋体", Font.PLAIN, 16));
textArea.addKeyListener(this);
JScrollPane scrollPane = new JScrollPane(textArea);
statusLabel = new JLabel("就绪");
statusLabel.setPreferredSize(new Dimension(200, 20));
contentPane.add(scrollPane, BorderLayout.CENTER);
contentPane.add(statusLabel, BorderLayout.SOUTH);
JMenuBar menuBar = new JMenuBar();
JMenu fileMenu = new JMenu("文件");
JMenuItem newMenuItem = new JMenuItem("新建");
newMenuItem.setAccelerator(KeyStroke.getKeyStroke(KeyEvent.VK_N, KeyEvent.CTRL_DOWN_MASK));
newMenuItem.addActionListener(this);
fileMenu.add(newMenuItem);
JMenuItem openMenuItem = new JMenuItem("打开");
openMenuItem.setAccelerator(KeyStroke.getKeyStroke(KeyEvent.VK_O, KeyEvent.CTRL_DOWN_MASK));
openMenuItem.addActionListener(this);
fileMenu.add(openMenuItem);
JMenuItem saveMenuItem = new JMenuItem("保存");
saveMenuItem.setAccelerator(KeyStroke.getKeyStroke(KeyEvent.VK_S, KeyEvent.CTRL_DOWN_MASK));
saveMenuItem.addActionListener(this);
fileMenu.add(saveMenuItem);
JMenuItem saveAsMenuItem = new JMenuItem("另存为");
saveAsMenuItem.addActionListener(this);
fileMenu.add(saveAsMenuItem);
menuBar.add(fileMenu);
JMenu editMenu = new JMenu("编辑");
JMenuItem copyMenuItem = new JMenuItem("复制");
copyMenuItem.setAccelerator(KeyStroke.getKeyStroke(KeyEvent.VK_C, KeyEvent.CTRL_DOWN_MASK));
copyMenuItem.addActionListener(this);
editMenu.add(copyMenuItem);
JMenuItem pasteMenuItem = new JMenuItem("粘贴");
pasteMenuItem.setAccelerator(KeyStroke.getKeyStroke(KeyEvent.VK_V, KeyEvent.CTRL_DOWN_MASK));
pasteMenuItem.addActionListener(this);
editMenu.add(pasteMenuItem);
JMenuItem findMenuItem = new JMenuItem("查找");
findMenuItem.setAccelerator(KeyStroke.getKeyStroke(KeyEvent.VK_F, KeyEvent.CTRL_DOWN_MASK));
findMenuItem.addActionListener(this);
editMenu.add(findMenuItem);
JMenuItem replaceMenuItem = new JMenuItem("替换");
replaceMenuItem.setAccelerator(KeyStroke.getKeyStroke(KeyEvent.VK_H, KeyEvent.CTRL_DOWN_MASK));
replaceMenuItem.addActionListener(this);
editMenu.add(replaceMenuItem);
menuBar.add(editMenu);
JMenu helpMenu = new JMenu("帮助");
JMenuItem aboutMenuItem = new JMenuItem("关于");
aboutMenuItem.addActionListener(this);
helpMenu.add(aboutMenuItem);
menuBar.add(Box.createHorizontalGlue());
menuBar.add(helpMenu);
setJMenuBar(menuBar);
JPanel buttonPanel = new JPanel(new FlowLayout(FlowLayout.RIGHT));
JButton newButton = new JButton("新建");
newButton.addActionListener(this);
buttonPanel.add(newButton);
JButton openButton = new JButton("打开");
openButton.addActionListener(this);
buttonPanel.add(openButton);
JButton saveButton = new JButton("保存");
saveButton.addActionListener(this);
buttonPanel.add(saveButton);
contentPane.add(buttonPanel, BorderLayout.NORTH);
setDefaultCloseOperation(WindowConstants.DO_NOTHING_ON_CLOSE);
addWindowListener(new java.awt.event.WindowAdapter() {
public void windowClosing(java.awt.event.WindowEvent evt) {
exitApplication();
}
});
pack();
setLocationRelativeTo(null);
setVisible(true);
}
public static void main(String[] args) {
new Notepad();
}
private void exitApplication() {
if (modified) {
int choice = JOptionPane.showConfirmDialog(this, "是否保存修改?", "记事本", JOptionPane.YES_NO_CANCEL_OPTION);
if (choice == JOptionPane.YES_OPTION) {
saveFile();
} else if (choice == JOptionPane.CANCEL_OPTION) {
return;
}
}
dispose();
System.exit(0);
}
private void newFile() {
if (modified) {
int choice = JOptionPane.showConfirmDialog(this, "是否保存修改?", "记事本", JOptionPane.YES_NO_CANCEL_OPTION);
if (choice == JOptionPane.YES_OPTION) {
saveFile();
} else if (choice == JOptionPane.CANCEL_OPTION) {
return;
}
}
textArea.setText("");
setTitle("无标题");
currentFile = null;
modified = false;
}
private void openFile() {
if (modified) {
int choice = JOptionPane.showConfirmDialog(this, "是否保存修改?", "记事本", JOptionPane.YES_NO_CANCEL_OPTION);
if (choice == JOptionPane.YES_OPTION) {
saveFile();
} else if (choice == JOptionPane.CANCEL_OPTION) {
return;
}
}
JFileChooser fileChooser = new JFileChooser();
int result = fileChooser.showOpenDialog(this);
if (result == JFileChooser.APPROVE_OPTION) {
File file = fileChooser.getSelectedFile();
try {
BufferedReader reader = new BufferedReader(new FileReader(file));
String line;
StringBuilder buffer = new StringBuilder();
while ((line = reader.readLine()) != null) {
buffer.append(line).append("\n");
}
reader.close();
textArea.setText(buffer.toString());
setTitle(file.getAbsolutePath());
currentFile = file;
modified = false;
} catch (IOException e) {
JOptionPane.showMessageDialog(this, e.getMessage(), "错误", JOptionPane.ERROR_MESSAGE);
}
}
}
private void saveFile() {
if (currentFile == null) {
JFileChooser fileChooser = new JFileChooser();
int result = fileChooser.showSaveDialog(this);
if (result == JFileChooser.APPROVE_OPTION) {
currentFile = fileChooser.getSelectedFile();
} else {
return;
}
}
try {
FileWriter writer = new FileWriter(currentFile);
writer.write(textArea.getText());
writer.close();
setTitle(currentFile.getAbsolutePath());
modified = false;
} catch (IOException e) {
JOptionPane.showMessageDialog(this, e.getMessage(), "错误", JOptionPane.ERROR_MESSAGE);
}
}
private void saveFileAs() {
JFileChooser fileChooser = new JFileChooser();
int result = fileChooser.showSaveDialog(this);
if (result == JFileChooser.APPROVE_OPTION) {
currentFile = fileChooser.getSelectedFile();
saveFile();
}
}
private void copyText() {
textArea.copy();
}
private void pasteText() {
textArea.paste();
}
private void findText() {
String text = JOptionPane.showInputDialog(this, "查找:", "记事本", JOptionPane.PLAIN_MESSAGE);
if (text != null) {
String content = textArea.getText();
int index = content.indexOf(text);
if (index == -1) {
JOptionPane.showMessageDialog(this, "未找到指定文本。", "记事本", JOptionPane.INFORMATION_MESSAGE);
} else {
textArea.select(index, index + text.length());
}
}
}
private void replaceText() {
String findText = JOptionPane.showInputDialog(this, "查找:", "记事本", JOptionPane.PLAIN_MESSAGE);
if (findText != null) {
String replaceText = JOptionPane.showInputDialog(this, "替换为:", "记事本", JOptionPane.PLAIN_MESSAGE);
if (replaceText != null) {
String content = textArea.getText();
int index = content.indexOf(findText);
if (index == -1) {
JOptionPane.showMessageDialog(this, "未找到指定文本。", "记事本", JOptionPane.INFORMATION_MESSAGE);
} else {
textArea.replaceRange(replaceText, index, index + findText.length());
modified = true;
}
}
}
}
private void about() {
JOptionPane.showMessageDialog(this, "记事本\n\n版本:1.0\n开发者:xxx\n日期:2022年1月1日", "关于", JOptionPane.INFORMATION_MESSAGE);
}
@Override
public void actionPerformed(ActionEvent e) {
String command = e.getActionCommand();
switch (command) {
case "新建":
case "新建(N)...":
case "Ctrl+N":
newFile();
break;
case "打开...":
case "打开(O)...":
case "Ctrl+O":
openFile();
break;
case "保存":
case "保存(S)":
case "Ctrl+S":
saveFile();
break;
case "另存为(A)...":
saveFileAs();
break;
case "复制":
case "复制(C)":
case "Ctrl+C":
copyText();
break;
case "粘贴":
case "粘贴(P)":
case "Ctrl+V":
pasteText();
break;
case "查找...":
case "查找(F)...":
case "Ctrl+F":
findText();
break;
case "替换...":
case "替换(R)...":
case "Ctrl+H":
replaceText();
break;
case "关于记事本":
about();
break;
}
}
@Override
public void keyPressed(KeyEvent e) {
if (e.getKeyCode() == KeyEvent.VK_ENTER || e.getKeyCode() == KeyEvent.VK_BACK_SPACE) {
modified = true;
}
}
@Override
public void keyTyped(KeyEvent e) {
}
@Override
public void keyReleased(KeyEvent e) {
int length = textArea.getText().length();
int caretPosition = textArea.getCaretPosition();
statusLabel.setText(String.format("第 %d 行,第 %d 列,共 %d 个字符", textArea.getLineOfOffset(caretPosition) + 1,
caretPosition - textArea.getLineStartOffset(textArea.getLineOfOffset(caretPosition)) + 1, length));
}
}
```
注意,这只是一个简单的实现,可能存在一些漏洞和不足之处,需要根据实际需求进行改进。
相关推荐
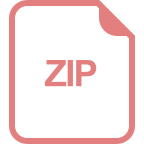
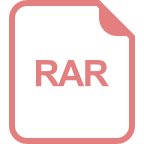













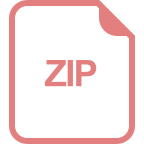
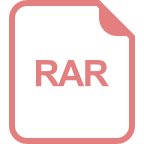