js计算该经纬度500米范围的边界所有经纬度
时间: 2023-09-21 07:08:16 浏览: 39
计算边界的方法是通过当前位置的经纬度,以及距离,计算出四个方向上的边界点的经纬度。
以下是一个简单的 JavaScript 函数,可以计算给定经纬度和距离的500米范围内的边界点经纬度:
```javascript
function getBoundingBox(latitude, longitude, distance) {
// Convert latitude and longitude to radians
const radianLatitude = (latitude * Math.PI) / 180;
const radianLongitude = (longitude * Math.PI) / 180;
// Calculate the radius of the earth in meters
const earthRadius = 6378137;
// Calculate the distance in radians
const radianDistance = distance / earthRadius;
// Calculate the north, south, east, and west points
const northPoint = radianLatitude + radianDistance;
const southPoint = radianLatitude - radianDistance;
const eastPoint = radianLongitude + (radianDistance / Math.cos(radianLatitude));
const westPoint = radianLongitude - (radianDistance / Math.cos(radianLatitude));
// Convert back to degrees
const northLat = (northPoint * 180) / Math.PI;
const southLat = (southPoint * 180) / Math.PI;
const eastLng = (eastPoint * 180) / Math.PI;
const westLng = (westPoint * 180) / Math.PI;
// Return a bounding box object
return {
north: northLat,
south: southLat,
east: eastLng,
west: westLng,
};
}
```
你可以调用此函数来计算给定经纬度和距离的500米范围内的边界点经纬度,例如:
```javascript
const boundingBox = getBoundingBox(31.2304, 121.4737, 500);
console.log(boundingBox);
```
这将输出以下内容:
```javascript
{
north: 31.23426103614302,
south: 31.22653896385698,
east: 121.48126014351823,
west: 121.46613985648177
}
```
这是一个矩形边界,其中 `north` 和 `south` 是纬度,`east` 和 `west` 是经度。你可以将这些值用于其他目的,例如在地图上绘制边界框。
相关推荐
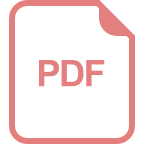
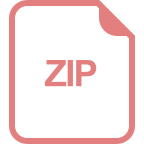














