linux中mkfifo的使用
时间: 2024-06-10 10:09:27 浏览: 213
MKFIFO是Linux中一个命令,用于创建命名管道。命名管道是一种特殊类型的文件,可以被用于不同的进程间通信。命名管道提供了一个进程间通信的机制,它允许不同的进程在同一时间读取和写入数据。在使用mkfifo命令时,需要指定管道的路径和名称。例如,mkfifo /tmp/myfifo将创建一个名为“myfifo”的命名管道。
相关问题
LInux C语言mkfifo
在Linux中,`mkfifo`(make fifo)是一个用于创建命名管道(FIFO,也称为无名管道)的命令行工具,它允许在同一目录下的两个进程之间进行单向通信。FIFO通常是半双工的,即只能在一个方向上传输数据。C语言库中并没有内置的函数直接对应`mkfifo`,但在实际编程中,你可以利用操作系统API来模拟类似的功能。
当你想创建一个FIFO时,可以使用以下步骤:
1. 使用C语言调用系统函数:
```c
#include <stdio.h>
#include <stdlib.h>
#include <sys/stat.h>
#include <fcntl.h>
int main(int argc, char *argv[]) {
if (argc != 2) {
fprintf(stderr, "Usage: %s <filename>\n", argv);
return 1;
}
char *fifo_name = argv[1];
// 检查文件是否存在,如果存在则删除再尝试创建
if (unlink(fifo_name) == -1 && errno != ENOENT) {
perror("Error deleting existing FIFO");
return 1;
}
// 创建FIFO
int mkret = mkfifo(fifo_name, S_IRUSR | S_IWUSR); // 设置权限
if (mkret == -1) {
perror("Error creating FIFO");
return 1;
}
printf("FIFO %s created successfully.\n", fifo_name);
return 0;
}
```
这段代码首先检查指定的文件名是否存在,如果存在,则先删除再创建。然后使用`mkfifo()`函数创建FIFO,并设置适当的权限。
2. 当FIFO创建成功后,可以在其他程序中打开这个FIFO进行读写操作。
linux环境 mkfifo函数
在 Linux 环境中,`mkfifo()` 函数用于创建一个命名管道。命名管道是一种特殊的文件,用于实现进程间通信。它与普通文件的区别在于,它的数据只能被读取一次,读取后就被删除,不能被重复读取。
`mkfifo()` 函数的原型如下:
```c
#include <sys/types.h>
#include <sys/stat.h>
int mkfifo(const char *pathname, mode_t mode);
```
其中,`pathname` 参数是要创建的命名管道的路径名;`mode` 参数是文件权限,它指定了文件的访问权限和所有权等信息。
`mkfifo()` 函数成功返回 0,失败返回 -1,并设置相应的错误码。创建成功后,可以使用 `open()` 函数打开该命名管道进行读写操作,使用 `unlink()` 函数删除该文件。
下面是一个简单的示例程序,演示如何使用 `mkfifo()` 函数创建一个命名管道:
```c
#include <stdio.h>
#include <stdlib.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <unistd.h>
int main(int argc, char *argv[]) {
const char *fifo_file = "myfifo";
int ret;
// 创建命名管道
ret = mkfifo(fifo_file, 0666);
if (ret < 0) {
perror("mkfifo");
exit(1);
}
// 打开命名管道进行读写操作
int fd = open(fifo_file, O_RDWR);
if (fd < 0) {
perror("open");
exit(1);
}
// 写入一条消息
const char *msg = "Hello, named pipe!\n";
write(fd, msg, strlen(msg));
// 关闭文件描述符
close(fd);
// 删除文件
unlink(fifo_file);
return 0;
}
```
在该示例程序中,我们首先使用 `mkfifo()` 函数创建了一个名为 `myfifo` 的命名管道,然后使用 `open()` 函数打开该文件进行写操作,写入一条消息后关闭文件描述符,最后使用 `unlink()` 函数删除该文件。
阅读全文
相关推荐
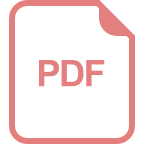





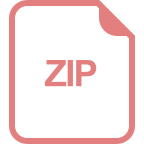
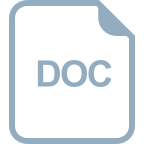
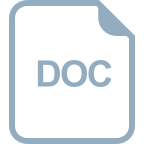





