def crop_pointcloud(data_crop, x_o, y_o, x_i, y_i, R_o, R_i, z_critical): K_o = R_o ** 2 / range_z K_i = R_i ** 2 / range_z for z in range(range_z): r_o = np.sqrt(z * K_o) data_layer = data_crop[:, :, z] d_o = np.sqrt(x_o ** 2 + y_o ** 2) d_i = np.sqrt(x_i ** 2 + y_i ** 2) if z < z_critical: r_i = 0 else: r_i = np.sqrt(z * K_i) data_crop[:, :, z] = np.where((d_o > r_o) | (d_i <= r_i), 0, data_layer) return data_crop data_crop = data[:, :, :400] print(file_path) # np.savetxt('reshape_data.txt', data_crop, delimiter=',') range_x, range_y, range_z = data_crop.shape x, y = np.meshgrid(np.arange(range_x), np.arange(range_y)) # np.savetxt('reshape_data.txt', x, delimiter=' ', fmt="%i") x_o = x - range_x / 2 y_o = y - range_y / 2 x_i = x - dx y_i = y - dy z_critical = 50 R_o = 550 R_i = 200 data_crop = crop_pointcloud(data_crop, x_o, y_o, x_i, y_i, R_o, R_i, z_critical) data_crop = data_crop[:, :, 10:] C++ Eigen::Tensor
时间: 2024-03-19 18:41:01 浏览: 14
Eigen::Tensor is a C++ library for tensor operations, developed by the Eigen team. It provides a high-level API for performing tensor operations, such as tensor addition, multiplication, and convolution. The library is designed to be fast and efficient, and provides support for both CPU and GPU acceleration. Eigen::Tensor is particularly useful for machine learning applications, where tensors are commonly used to represent data.
相关问题
def crop_pointcloud(data_crop, x_o, y_o, x_i, y_i, R_o, R_i, z_critical): K_o = R_o ** 2 / range_z K_i = R_i ** 2 / range_z for z in range(range_z): r_o = np.sqrt(z * K_o) data_layer = data_crop[:, :, z] d_o = np.sqrt(x_o ** 2 + y_o ** 2) d_i = np.sqrt(x_i ** 2 + y_i ** 2) if z < z_critical: r_i = 0 else: r_i = np.sqrt(z * K_i) data_crop[:, :, z] = np.where((d_o > r_o) | (d_i <= r_i), 0, data_layer) return data_crop data_crop = data[:, :, :400] range_x, range_y, range_z = data_crop.shape dx = 550 dy = 530 x, y = np.meshgrid(np.arange(range_x), np.arange(range_y)) x_o = x - range_x / 2 y_o = y - range_y / 2 x_i = x - dx y_i = y - dy z_critical = 50 R_o = 550 R_i = 200 data_crop = crop_pointcloud(data_crop, x_o, y_o, x_i, y_i, R_o, R_i, z_critical) data_crop = data_crop[:, :, 10:]转c++
这段 Python 代码主要是对点云数据进行裁剪,并返回裁剪后的点云数据。以下是将其转换为 C++ 的代码:
```cpp
#include <cmath>
#include <vector>
#include <algorithm>
void crop_pointcloud(std::vector<std::vector<std::vector<float>>>& data_crop,
int x_o, int y_o, int x_i, int y_i, int R_o, int R_i, int z_critical)
{
int range_x = data_crop.size();
int range_y = data_crop[0].size();
int range_z = data_crop[0][0].size();
float K_o = std::pow(R_o, 2) / range_z;
float K_i = std::pow(R_i, 2) / range_z;
for (int z = 0; z < range_z; ++z) {
float r_o = std::sqrt(z * K_o);
std::vector<std::vector<float>>& data_layer = data_crop[z];
float d_o = std::sqrt(std::pow(x_o, 2) + std::pow(y_o, 2));
float d_i = std::sqrt(std::pow(x_i, 2) + std::pow(y_i, 2));
float r_i = (z < z_critical) ? 0 : std::sqrt(z * K_i);
for (int i = 0; i < range_x; ++i) {
for (int j = 0; j < range_y; ++j) {
if (d_o > r_o || d_i <= r_i) {
data_layer[i][j] = 0;
}
}
}
}
}
int main()
{
std::vector<std::vector<std::vector<float>>> data_crop; // 假设已经读入了点云数据
int range_x = data_crop.size();
int range_y = data_crop[0].size();
int range_z = data_crop[0][0].size();
int dx = 550;
int dy = 530;
int x_o, y_o, x_i, y_i;
x_o = x_i = range_x / 2;
y_o = y_i = range_y / 2;
int z_critical = 50;
int R_o = 550;
int R_i = 200;
crop_pointcloud(data_crop, x_o, y_o, x_i, y_i, R_o, R_i, z_critical);
// 剩余部分请自行完成
return 0;
}
```
需要注意的是,在 C++ 中使用多维数组需要使用嵌套的 `std::vector`,因此在 C++ 的实现中需要对数组的访问进行适当修改。此外,需要将 `numpy` 的一些函数,比如 `np.arange` 和 `np.where` 等等,转换为 C++ 中的等价函数。
x, y = np.meshgrid(np.arange(range_x), np.arange(range_y)) # np.savetxt('reshape_data.txt', x, delimiter=' ', fmt="%i") x_o = x - range_x / 2 y_o = y - range_y / 2 x_i = x - dx y_i = y - dy z_critical = 50 R_o = 550 R_i = 200 def crop_pointcloud(data_crop, x_o, y_o, x_i, y_i, R_o, R_i, z_critical): K_o = R_o ** 2 / range_z K_i = R_i ** 2 / range_z for z in range(range_z): r_o = np.sqrt(z * K_o) data_layer = data_crop[:, :, z] d_o = np.sqrt(x_o ** 2 + y_o ** 2) d_i = np.sqrt(x_i ** 2 + y_i ** 2) if z < z_critical: r_i = 0 else: r_i = np.sqrt(z * K_i) data_crop[:, :, z] = np.where((d_o > r_o) | (d_i <= r_i), 0, data_layer) return data_crop data_crop = crop_pointcloud(data_crop, x_o, y_o, x_i, y_i, R_o, R_i, z_critical) data_crop = data_crop[:, :, 10:] C++ Eigen::Tensor实现
以下是使用C++ Eigen::Tensor实现上述代码的示例:
```cpp
#include <iostream>
#include <Eigen/Dense>
#include <unsupported/Eigen/CXX11/Tensor>
using namespace Eigen;
template<typename T>
void crop_pointcloud(Tensor<T, 3>& data_crop, const Tensor<T, 2>& x_o, const Tensor<T, 2>& y_o, const Tensor<T, 2>& x_i, const Tensor<T, 2>& y_i, const T& R_o, const T& R_i, const int& z_critical)
{
const int range_z = data_crop.dimension(2);
const T K_o = R_o * R_o / range_z;
const T K_i = R_i * R_i / range_z;
for (int z = 0; z < range_z; ++z)
{
const T r_o = std::sqrt(z * K_o);
auto data_layer = data_crop.chip(z, 2);
const auto d_o = (x_o.square() + y_o.square()).sqrt();
const auto d_i = (x_i.square() + y_i.square()).sqrt();
const T r_i = (z < z_critical) ? T(0) : std::sqrt(z * K_i);
data_crop.chip(z, 2) = (d_o > r_o || d_i <= r_i).select(T(0), data_layer);
}
}
int main()
{
const int range_x = 100;
const int range_y = 100;
const int range_z = 100;
Tensor<float, 3> data_crop(range_x, range_y, range_z);
Tensor<int, 2> x(range_x, range_y);
Tensor<int, 2> y(range_x, range_y);
for (int i = 0; i < range_x; ++i)
{
for (int j = 0; j < range_y; ++j)
{
x(i, j) = i;
y(i, j) = j;
for (int k = 0; k < range_z; ++k)
{
data_crop(i, j, k) = i + j + k; // 假设这是点云数据
}
}
}
Tensor<float, 2> x_o = x.cast<float>() - range_x / 2;
Tensor<float, 2> y_o = y.cast<float>() - range_y / 2;
Tensor<float, 2> x_i = x.cast<float>() - 1; // 假设dx和dy都是1
Tensor<float, 2> y_i = y.cast<float>() - 1;
const float R_o = 550;
const float R_i = 200;
const int z_critical = 50;
crop_pointcloud(data_crop, x_o, y_o, x_i, y_i, R_o, R_i, z_critical);
data_crop = data_crop.slice({ 0, 0, 10 }, { range_x, range_y, range_z - 10 });
std::cout << data_crop << std::endl; // 输出处理后的点云数据
return 0;
}
```
这里使用了C++11的`auto`关键字和`Tensor`类来简化代码,并且使用了`slice`函数来去掉处理后的点云数据的前10层。
相关推荐
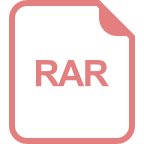
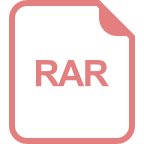

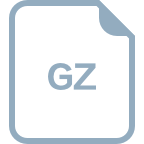
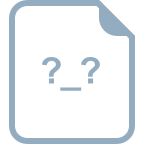
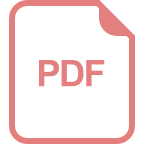
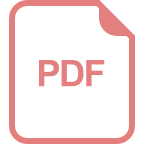
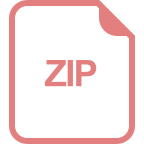