用python编写一个程序,用于对C源程序进行括号匹配检查,并将检查结果输出到文件中。 程序应检查输入源程序中左右大括号、小括号的个数是否相等,并且符合C语言语法规定的括号嵌套规则,不得交叉嵌套。输入文件最长不超过500个字符。 本程序只检查括号是否匹配,不用对输入源程序中的其它语法成份进行检查
时间: 2023-11-14 22:09:47 浏览: 40
以下是一个用Python编写的括号匹配检查程序:
```python
def check_brackets(input_file_path, output_file_path):
with open(input_file_path, 'r') as input_file, open(output_file_path, 'w') as output_file:
content = input_file.readline().strip()
stack = []
error = False
for i, char in enumerate(content):
if char in ['(', '[', '{']:
stack.append(char)
elif char in [')', ']', '}']:
if not stack:
output_file.write(f'Error: unmatched {char} at position {i+1}\n')
error = True
continue
top = stack.pop()
if (top == '(' and char != ')') or (top == '[' and char != ']') or (top == '{' and char != '}'):
output_file.write(f'Error: mismatched {top} and {char} at position {i+1}\n')
error = True
if stack:
output_file.write(f'Error: unmatched {stack[-1]} at position {len(content)}\n')
error = True
if not error:
output_file.write('Brackets match\n')
```
该程序使用了栈来检查括号匹配,对于左括号,将其压入栈中;对于右括号,弹出栈顶元素并检查是否匹配。如果栈为空或者括号不匹配,则输出错误信息。最后检查栈是否为空,如果不为空,则说明有未匹配的左括号。如果没有错误,则输出“Brackets match”。
要使用该程序,只需调用`check_brackets`函数,传入输入文件路径和输出文件路径即可。
```python
check_brackets('input.c', 'output.txt')
```
其中,`input.c`是输入源程序文件,`output.txt`是输出文件。输出文件中会显示括号匹配情况,如果有错误,则会显示错误信息。
相关推荐
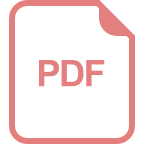
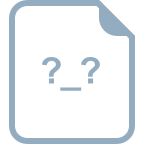









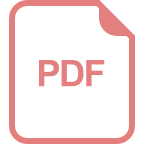
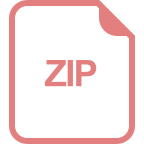
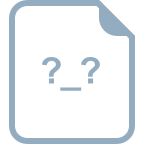
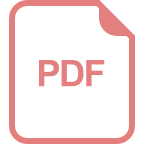