springboot+vue实现首页轮播图并在另一页面对轮播图进行增删改查后首页轮播图更新的代码
时间: 2024-02-01 16:02:22 浏览: 165
以下是使用Spring Boot和Vue.js实现首页轮播图并在另一页面对轮播图进行增删改查后首页轮播图更新的代码示例:
1. 后端代码(使用Spring Boot):
```java
// 轮播图实体类
@Entity
public class Carousel {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String imageUrl;
// 其他属性...
// 省略getter和setter方法
}
// 轮播图控制器
@RestController
@RequestMapping("/carousel")
public class CarouselController {
@Autowired
private CarouselRepository carouselRepository;
// 获取所有轮播图
@GetMapping("/")
public List<Carousel> getAllCarousels() {
return carouselRepository.findAll();
}
// 添加轮播图
@PostMapping("/")
public Carousel addCarousel(@RequestBody Carousel carousel) {
return carouselRepository.save(carousel);
}
// 删除轮播图
@DeleteMapping("/{id}")
public void deleteCarousel(@PathVariable Long id) {
carouselRepository.deleteById(id);
}
// 更新轮播图
@PutMapping("/{id}")
public Carousel updateCarousel(@PathVariable Long id, @RequestBody Carousel carousel) {
carousel.setId(id);
return carouselRepository.save(carousel);
}
}
// 轮播图仓库
public interface CarouselRepository extends JpaRepository<Carousel, Long> {
}
```
2. 前端代码(使用Vue.js和Element UI):
```vue
<template>
<div>
<!-- 首页轮播图 -->
<el-carousel :interval="5000">
<el-carousel-item v-for="carousel in carousels" :key="carousel.id">
<img :src="carousel.imageUrl" alt="carousel image">
</el-carousel-item>
</el-carousel>
<!-- 轮播图管理页面 -->
<el-table :data="carousels" style="margin-top: 20px;">
<el-table-column prop="imageUrl" label="图片URL"></el-table-column>
<!-- 其他列... -->
<el-table-column label="操作">
<template slot-scope="scope">
<el-button type="primary" size="small" @click="editCarousel(scope.row)">编辑</el-button>
<el-button type="danger" size="small" @click="deleteCarousel(scope.row)">删除</el-button>
</template>
</el-table-column>
</el-table>
</div>
</template>
<script>
export default {
data() {
return {
carousels: [] // 轮播图数据
};
},
mounted() {
this.getCarousels(); // 页面加载时获取轮播图数据
},
methods: {
// 获取轮播图数据
getCarousels() {
// 发送Ajax请求获取轮播图数据
axios.get('/carousel/')
.then(response => {
this.carousels = response.data;
})
.catch(error => {
console.error(error);
});
},
// 添加轮播图
addCarousel() {
// 发送Ajax请求添加轮播图
axios.post('/carousel/', { imageUrl: '轮播图URL' })
.then(response => {
this.getCarousels(); // 添加成功后刷新轮播图数据
})
.catch(error => {
console.error(error);
});
},
// 编辑轮播图
editCarousel(carousel) {
// 发送Ajax请求更新轮播图
axios.put(`/carousel/${carousel.id}`, carousel)
.then(response => {
this.getCarousels(); // 更新成功后刷新轮播图数据
})
.catch(error => {
console.error(error);
});
},
// 删除轮播图
deleteCarousel(carousel) {
// 发送Ajax请求删除轮播图
axios.delete(`/carousel/${carousel.id}`)
.then(response => {
this.getCarousels(); // 删除成功后刷新轮播图数据
})
.catch(error => {
console.error(error);
});
}
}
};
</script>
```
相关推荐
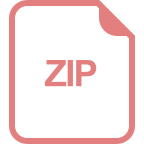
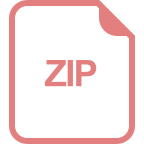














