用DVE-C++写出二叉树的顺序存储代码
时间: 2024-10-20 15:19:45 浏览: 35
在使用DVE-C++(一种C++库用于数据可视化)创建二叉树的顺序存储实现时,我们通常会使用数组来存储节点的信息,因为顺序存储方式适合于线性结构。首先,我们需要定义一个二叉树节点的数据结构,并将其存储在一个动态数组中。
```cpp
#include <vector>
using namespace DVE;
// 定义二叉树节点
struct TreeNode {
int data;
TreeNode* left;
TreeNode* right;
};
// 动态数组用于顺序存储二叉树
std::vector<TreeNode*> binaryTreeStorage;
// 插入节点函数,这里仅作演示,实际应用需要考虑平衡和空间管理
void insertNode(TreeNode** storage, int index, int value) {
if (index >= storage->size()) {
(*storage)[index] = new TreeNode{value, nullptr, nullptr};
} else {
(*storage)[index].data = value; // 如果索引已存在,则更新值
}
}
// 示例:创建并插入节点
void buildSequentialBinaryTree() {
TreeNode* root = new TreeNode{1, nullptr, nullptr}; // 根节点
binaryTreeStorage.push_back(root);
// 添加其他节点...
insertNode(&binaryTreeStorage, 0, 2); // 左孩子
insertNode(&binaryTreeStorage, 1, 3); // 右孩子
// 更复杂的层次添加...
// ... 具体的递归插入或循环遍历算法实现细节省略
}
阅读全文
相关推荐
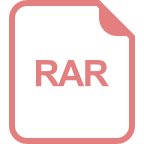
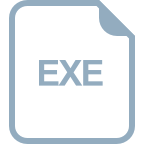

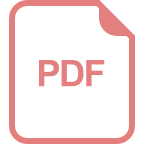
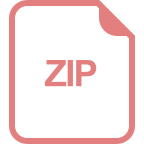

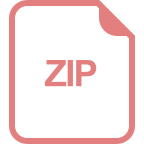
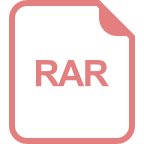


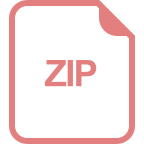
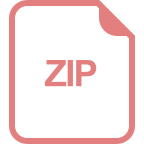
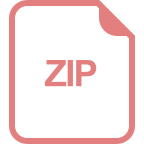
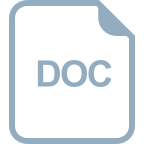


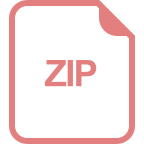