怎么使用Yolo算法提取视频中的车流量数据,代码举例。
时间: 2024-09-21 21:09:34 浏览: 28
YOLO (You Only Look Once) 算法是一种实时目标检测模型,常用于行人、车辆等物体的识别。要在视频中提取车流量数据,首先需要安装必要的库(如TensorFlow、OpenCV、Pillow等),然后按照以下步骤操作:
1. **安装依赖**:
```bash
pip install tensorflow opencv-python Pillow
```
2. **预处理视频**:
使用OpenCV读取视频并将其转换成YOLO所需的输入格式。例如:
```python
import cv2
cap = cv2.VideoCapture('video.mp4')
width = int(cap.get(cv2.CAP_PROP_FRAME_WIDTH))
height = int(cap.get(cv2.CAP_PROP_FRAME_HEIGHT))
# 如果需要调整YOLO的输入大小,可以在这里设置
input_size = 416
dim = (input_size, input_size)
```
3. **加载YOLO模型**:
```python
from yolov5.yolov5 import YOLOv5
yolo = YOLOv5(weights='yolov5s.pt', device='cuda') if torch.cuda.is_available() else YOLOv5(weights='yolov5s.yaml')
```
4. **循环检测帧并计数**:
```python
car_count = 0
while True:
ret, frame = cap.read()
if not ret:
break
img = letterbox(frame, new_shape=dim)[0]
img = img[:, :, ::-1].transpose(2, 0, 1) # BGR to RGB, HWC to CHW
img = np.ascontiguousarray(img)
results = yolo(img)
boxes = results.xyxy[results.probability > threshold] # Apply detection threshold
for box in boxes:
x1, y1, x2, y2 = box.tolist()
label = yolo.names[int(box[5])]
if label == 'car':
car_count += 1
# 可能会添加车辆框可视化或其他统计分析
print(f"Frame: {cap.get(cv2.CAP_PROP_POS_FRAMES)} - Cars: {car_count}")
```
5. **关闭资源**:
```python
cap.release()
```
注意,这只是一个基础示例,实际应用可能需要对结果进行后处理(比如去除重复检测、跟踪等),并且阈值(`threshold`)需要适当调整以达到理想的精度和速度。
相关推荐
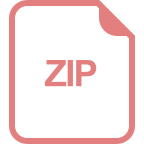
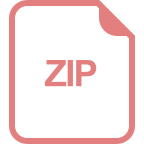
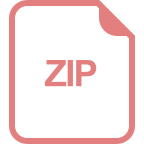














