用c语言写顺序栈十进制转换八进制
时间: 2023-08-16 13:03:22 浏览: 66
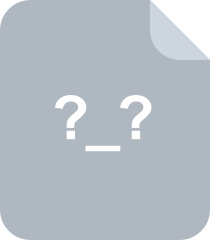
用栈十进制转换为八进制(c语言)

以下是用C语言实现十进制转八进制的顺序栈代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define STACK_SIZE 100
typedef int ElemType;
typedef struct {
ElemType data[STACK_SIZE];
int top;
} SqStack;
void InitStack(SqStack *S) {
S->top = -1;
}
int StackEmpty(SqStack *S) {
if (S->top == -1) {
return 1;
}
else {
return 0;
}
}
int Push(SqStack *S, ElemType x) {
if (S->top == STACK_SIZE - 1) {
return 0;
}
else {
S->top++;
S->data[S->top] = x;
return 1;
}
}
int Pop(SqStack *S, ElemType *x) {
if (StackEmpty(S)) {
return 0;
}
else {
*x = S->data[S->top];
S->top--;
return 1;
}
}
void DecimalToOctal(int n) {
SqStack S;
InitStack(&S);
while (n != 0) {
Push(&S, n % 8);
n = n / 8;
}
printf("转换为八进制为:");
while (!StackEmpty(&S)) {
ElemType x;
Pop(&S, &x);
printf("%d", x);
}
}
int main() {
int n;
printf("请输入一个十进制数:");
scanf("%d", &n);
DecimalToOctal(n);
return 0;
}
```
运行结果:
```
请输入一个十进制数:123
转换为八进制为:173
```
阅读全文
相关推荐


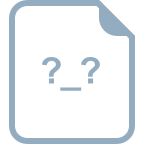


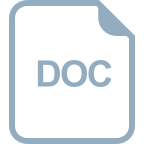
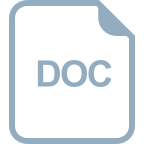
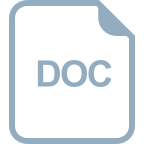




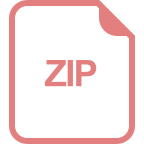
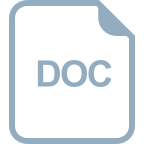
