写出一个arduino程序,该程序实现点唱机的切换音乐功能,该点唱机使用了sd卡模块,1602A显示屏,arduino开发板,扬声器等
时间: 2024-03-24 15:37:31 浏览: 89
好的,下面是一个简单的 Arduino 程序,可以实现基本的点唱机切换音乐功能:
```C++
#include <SD.h>
#include <LiquidCrystal.h>
// SD 卡模块的引脚定义
const int chipSelect = 4;
// LCD 显示屏的引脚定义
LiquidCrystal lcd(6, 7, 8, 9, 10, 11);
// 扬声器的引脚定义
const int speakerPin = 12;
// 音乐列表,假设 SD 卡根目录下有两首音乐文件 music1.mp3 和 music2.mp3
const char* musicList[] = {"music1.mp3", "music2.mp3"};
int currentMusicIndex = 0;
void setup() {
// 初始化 SD 卡模块
if (!SD.begin(chipSelect)) {
lcd.print("SD 卡初始化失败!");
return;
}
// 初始化 LCD 显示屏
lcd.begin(16, 2);
lcd.print("点唱机");
// 初始化扬声器
pinMode(speakerPin, OUTPUT);
}
void loop() {
// 播放当前选中的音乐
playMusic(musicList[currentMusicIndex]);
// 等待用户按下按钮,切换下一首音乐
while (true) {
lcd.setCursor(0, 1);
lcd.print("当前音乐:");
lcd.print(musicList[currentMusicIndex]);
delay(1000);
if (digitalRead(2) == LOW) { // 假设按钮连接到引脚 2
currentMusicIndex++;
if (currentMusicIndex >= sizeof(musicList) / sizeof(musicList[0])) {
currentMusicIndex = 0;
}
break;
}
}
}
void playMusic(const char* fileName) {
// 打开文件
File file = SD.open(fileName);
if (!file) {
lcd.print("文件打开失败!");
return;
}
// 播放音乐
while (file.available()) {
int data = file.read();
analogWrite(speakerPin, data);
}
// 关闭文件
file.close();
}
```
这个程序假设 SD 卡根目录下有两首音乐文件 music1.mp3 和 music2.mp3。程序会先播放当前选中的音乐,然后等待用户按下按钮,切换下一首音乐。在 LCD 显示屏上会显示当前选中的音乐文件名。注意,这个程序只是一个简单的示例,实际的点唱机功能可能更加复杂,需要根据具体需求进行修改和扩展。
阅读全文
相关推荐
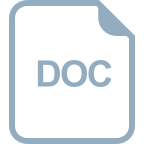
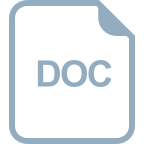
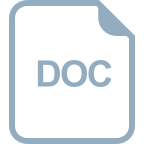



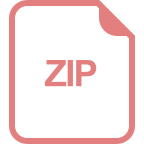
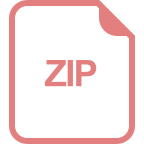
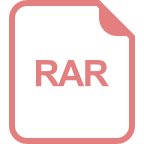
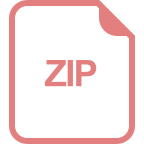
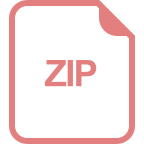
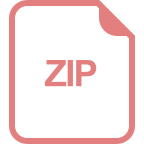
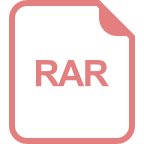
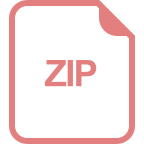