C# 加载透明背景
时间: 2023-07-12 11:12:04 浏览: 48
在 C# 中加载透明背景图片需要注意以下几点:
1. 要使用支持透明通道的图片格式,如 PNG 格式。
2. 在加载图片时,需要将图片的像素格式设置为 32 位,否则无法显示透明效果。
下面是代码示例:
```csharp
using System.Drawing;
// 加载 PNG 格式的透明背景图片
Bitmap bitmap = new Bitmap("transparentImage.png");
// 设置图片的像素格式为 32 位
bitmap = bitmap.Clone(new Rectangle(0, 0, bitmap.Width, bitmap.Height), PixelFormat.Format32bppArgb);
// 将图片显示在 PictureBox 控件中
pictureBox.Image = bitmap;
```
在以上示例中,我们首先使用 Bitmap 类加载 PNG 格式的透明背景图片,然后将图片的像素格式设置为 32 位,最后将图片显示在 PictureBox 控件中。这样就可以正确地显示透明背景了。
相关问题
C# 判断图片背景是否透明
可以通过C#中的System.Drawing.Imaging命名空间中的Image和Bitmap类来判断图片背景是否透明。具体实现方法如下:
1. 加载图片到Bitmap对象中:
```csharp
Bitmap bmp = new Bitmap("image.png");
```
2. 判断图片的PixelFormat是否为Format32bppArgb:
```csharp
if (bmp.PixelFormat == PixelFormat.Format32bppArgb)
{
// 图片背景为透明
}
else
{
// 图片背景不透明
}
```
其中,PixelFormat为枚举类型,包含了许多不同的像素格式,Format32bppArgb表示32位的像素格式,其中的Alpha通道用于表示透明度。
3. 释放Bitmap对象:
```csharp
bmp.Dispose();
```
最后需要记得释放Bitmap对象,以免出现内存泄漏等问题。
C# 将透明背景的bitmap设置为白色背景
你可以使用以下代码将透明背景的 Bitmap 设置为白色背景:
```csharp
Bitmap bmp = new Bitmap("image.png");
Bitmap newBmp = new Bitmap(bmp.Width, bmp.Height);
Graphics g = Graphics.FromImage(newBmp);
g.Clear(Color.White);
g.DrawImageUnscaled(bmp, 0, 0);
g.Dispose();
bmp.Dispose();
// 现在 newBmp 就是带有白色背景的 Bitmap
```
首先,你需要加载原始的透明背景 Bitmap。然后,创建一个新的 Bitmap,它的大小与原始 Bitmap 相同。接着,使用 Graphics 类的 Clear 方法将新 Bitmap 的背景设置为白色。最后,使用 Graphics 类的 DrawImageUnscaled 方法将原始 Bitmap 绘制到新 Bitmap 上。记得释放 Graphics 和原始 Bitmap 的资源。现在,新的 Bitmap 就带有白色背景了。
相关推荐
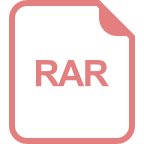








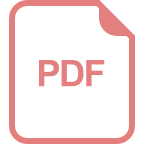
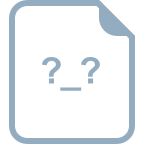
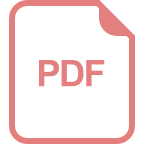
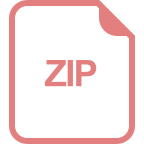
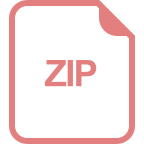