Write a program with the following requirements: 1. Use a 5x5 boolean two-dimensional array to represent the connectivity of the graphs that need to be traversed. 2. Use depth first algorithm to traverse the graph. 3. Use breadth first algorithm to traverse the graph. 4. The starting position of the traversal is chosen reasonably based on the connectivity of the graph.
时间: 2024-02-06 10:02:49 浏览: 103
Alright, I understand your requirements. Here's a Python program that satisfies your specifications:
```python
import queue
# Define the 5x5 boolean two-dimensional array to represent the connectivity of the graphs
graph = [
[False, True, False, True, False],
[True, False, True, False, True],
[False, True, False, True, False],
[True, False, True, False, True],
[False, True, False, True, False],
]
# Define the depth first traversal function
def depth_first_traversal(graph, start):
visited = [False] * len(graph)
stack = [start]
while stack:
vertex = stack.pop()
if not visited[vertex]:
print(vertex, end=" ")
visited[vertex] = True
for i in range(len(graph)):
if graph[vertex][i] and not visited[i]:
stack.append(i)
# Define the breadth first traversal function
def breadth_first_traversal(graph, start):
visited = [False] * len(graph)
q = queue.Queue()
q.put(start)
while not q.empty():
vertex = q.get()
if not visited[vertex]:
print(vertex, end=" ")
visited[vertex] = True
for i in range(len(graph)):
if graph[vertex][i] and not visited[i]:
q.put(i)
# Choose a reasonable starting position for the traversal based on the connectivity of the graph
start = 0
for i in range(len(graph)):
if sum(graph[i]) > sum(graph[start]):
start = i
# Perform depth first traversal
print("Depth First Traversal:")
depth_first_traversal(graph, start)
# Perform breadth first traversal
print("\nBreadth First Traversal:")
breadth_first_traversal(graph, start)
```
In this program, we first define the 5x5 boolean two-dimensional array `graph` to represent the connectivity of the graphs that need to be traversed. Then, we define the `depth_first_traversal` function and the `breadth_first_traversal` function to perform depth first and breadth first traversal of the graph respectively.
Next, we choose a reasonable starting position for the traversal based on the connectivity of the graph. We do this by iterating over each vertex in the graph and comparing the number of its neighbors to the number of neighbors of the current starting vertex. We choose the vertex with the highest number of neighbors as the starting position.
Finally, we call the `depth_first_traversal` function and the `breadth_first_traversal` function with the `graph` and `start` arguments to perform the traversals. The output of the program will be the sequence of vertices visited during the traversals.
阅读全文
相关推荐
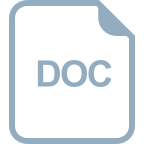
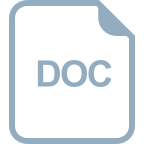
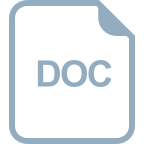
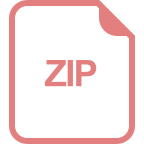
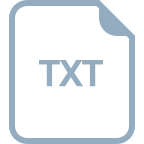
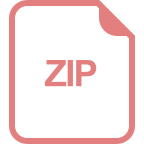
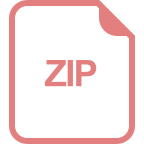
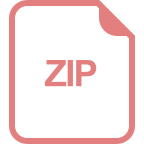
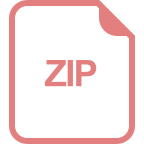
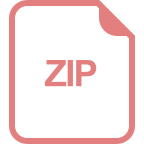
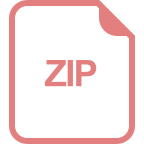
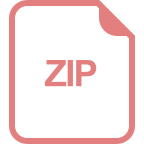
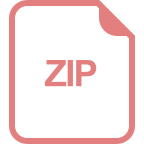
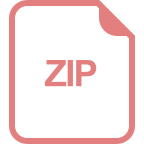
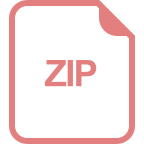
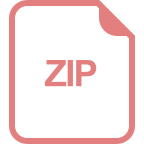
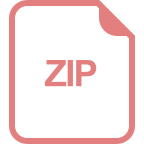
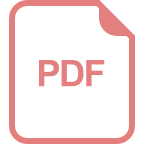
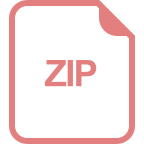