Entries in a stack are "ordered". What is the meaning of this statement?
时间: 2024-05-22 15:13:00 浏览: 12
The statement "entries in a stack are ordered" means that the elements or items in a stack are arranged in a specific manner, usually in a last-in-first-out (LIFO) manner, where the most recently added item is the first one to be removed. This ordering is essential to the functioning of a stack, as it ensures that items are removed in the correct order and that the integrity of the data structure is maintained.
相关问题
*:memcpy*.o(.text*) *:lib_a-memcpy*.o(.text*) *:libc_rom.o(.text*) *:libc_rom_mem.o(.text*) what is the function of after memcpy?
The entries that you listed are linker script commands that specify which sections of object files should be included in the final output executable.
In this case, the commands are specifying that any sections in object files with names matching `memcpy.o`, `lib_a-memcpy.o`, `libc_rom.o`, and `libc_rom_mem.o` that have names starting with `.text` should be included in the `.text` section of the final executable.
This means that any functions or code in those sections will be included in the final executable and can be executed by the program. `memcpy()` is a standard C library function that is used to copy a block of memory from one location to another, and it is likely that the object files with names matching `memcpy.o` or `lib_a-memcpy.o` contain the implementation of this function. The other object files may contain other C library functions or code that is required by the program.
how to input the adjacency matrix of a digraph to judge whether it is a simple digraph.
To input the adjacency matrix of a digraph and judge whether it is a simple digraph, follow these steps:
1. Write down the adjacency matrix of the digraph. The adjacency matrix is a square matrix where the entry in row i and column j represents the presence (or absence) of a directed edge from vertex i to vertex j. If there is an edge from i to j, the entry is 1, otherwise it is 0.
2. Check if there are any loops in the digraph. A loop is an edge that starts and ends at the same vertex. If there are any entries on the diagonal of the adjacency matrix that are non-zero, then there are loops present in the digraph.
3. Check if there are any multiple edges between two vertices. If there are any entries in the adjacency matrix that are greater than 1, then there are multiple edges between the corresponding vertices.
4. If there are no loops or multiple edges in the digraph, then it is a simple digraph. Otherwise, it is not a simple digraph.
Example: Consider the following adjacency matrix for a digraph:
0 1 0
1 0 1
0 1 0
This matrix represents a digraph with three vertices and three directed edges. There are no loops or multiple edges in this digraph, so it is a simple digraph.
相关推荐
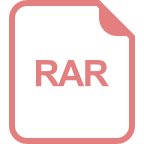
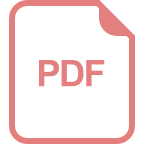












