如何用opencv和pytorch写一个简单的姿态识别代码
时间: 2024-05-10 18:18:52 浏览: 17
下面是一个简单的基于 OpenCV 和 PyTorch 的姿态识别代码,用于识别人体的躯干和四肢的轮廓,并预测其姿态:
```python
import cv2
import torch
import argparse
import numpy as np
from models import PoseEstimationModel
# 定义命令行参数
parser = argparse.ArgumentParser()
parser.add_argument('--model_path', type=str, default='models/pose_estimation_model.pt',
help='path to the pytorch pose estimation model')
parser.add_argument('--video_path', type=str, default='data/test_video.mp4',
help='path to the input video file')
args = parser.parse_args()
# 加载 PyTorch 模型
model = PoseEstimationModel()
model.load_state_dict(torch.load(args.model_path))
model.eval()
# 打开视频文件
cap = cv2.VideoCapture(args.video_path)
while cap.isOpened():
# 读取视频帧
ret, frame = cap.read()
if not ret:
break
# 将图像转换为 PyTorch Tensor
input_image = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
input_image = cv2.resize(input_image, (256, 256))
input_image = np.transpose(input_image, (2, 0, 1))
input_tensor = torch.tensor(input_image, dtype=torch.float32).unsqueeze(0)
# 前向传递,预测姿态
with torch.no_grad():
output = model(input_tensor)
keypoints = output.squeeze(0).numpy()
# 绘制姿态关键点
for i in range(0, len(keypoints), 3):
x, y, confidence = keypoints[i:i+3]
if confidence > 0.5:
cv2.circle(frame, (int(x), int(y)), 3, (0, 255, 0), -1)
# 显示图像
cv2.imshow('Pose Estimation', frame)
if cv2.waitKey(25) & 0xFF == ord('q'):
break
# 释放资源
cap.release()
cv2.destroyAllWindows()
```
注意,这段代码需要引入一个名为 `PoseEstimationModel` 的模型类,该类应该实现姿态估计模型的前向传递。您可以根据自己的需求来编写该类。此外,还需要在 `args.model_path` 中指定您的 PyTorch 模型的路径,以及在 `args.video_path` 中指定输入视频文件的路径。
相关推荐
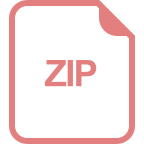
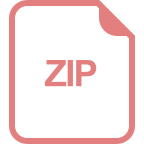
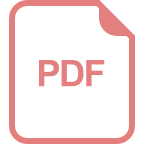














