java推箱子源代码
时间: 2023-08-31 21:06:02 浏览: 111
以下是一个简单的Java推箱子游戏的源代码实现:
```
import java.util.Scanner;
public class BoxPushGame {
// 地图的行数和列数
private static final int ROWS = 8;
private static final int COLS = 8;
// 地图数组
private static char[][] map = new char[ROWS][COLS];
// 玩家的位置
private static int playerRow = 0;
private static int playerCol = 0;
// 目标位置的数量
private static int targets = 0;
// 初始化地图
public static void initMap() {
String[] data = {
"########",
"# #",
"# #",
"# $ #",
"# @ #",
"# #",
"# #",
"########"
};
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
map[i][j] = data[i].charAt(j);
if (map[i][j] == '@') {
playerRow = i;
playerCol = j;
} else if (map[i][j] == '$') {
targets++;
}
}
}
}
// 输出地图
public static void printMap() {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
System.out.print(map[i][j]);
}
System.out.println();
}
}
// 移动玩家
public static boolean movePlayer(int row, int col) {
if (map[row][col] == '#') {
return false;
}
if (map[row][col] == '$') {
int dx = row - playerRow;
int dy = col - playerCol;
if (map[row+dx][col+dy] == '#' || map[row+dx][col+dy] == '$') {
return false;
}
map[row+dx][col+dy] = '$';
map[row][col] = ' ';
targets--;
}
map[playerRow][playerCol] = ' ';
map[row][col] = '@';
playerRow = row;
playerCol = col;
return true;
}
// 判断是否完成游戏
public static boolean isGameFinished() {
return targets == 0;
}
// 主函数
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
initMap();
while (true) {
printMap();
if (isGameFinished()) {
System.out.println("Congratulations! You win!");
break;
}
System.out.print("Please enter your move (up/down/left/right): ");
String input = scanner.nextLine();
int dx = 0;
int dy = 0;
if (input.equalsIgnoreCase("up")) {
dx = -1;
} else if (input.equalsIgnoreCase("down")) {
dx = 1;
} else if (input.equalsIgnoreCase("left")) {
dy = -1;
} else if (input.equalsIgnoreCase("right")) {
dy = 1;
}
if (dx != 0 || dy != 0) {
int newRow = playerRow + dx;
int newCol = playerCol + dy;
if (movePlayer(newRow, newCol)) {
System.out.println("Move successful.");
} else {
System.out.println("Move failed.");
}
}
}
}
}
```
这个程序使用一个二维字符数组来表示地图,其中 '#' 表示墙,' ' 表示空地,'$' 表示目标位置,'@' 表示玩家位置。玩家可以使用上下左右键来移动,如果玩家移动到目标位置上,则该目标位置会被清空。当所有目标位置都被清空后,玩家胜利。
阅读全文
相关推荐

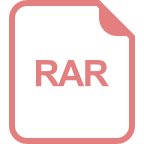

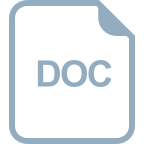


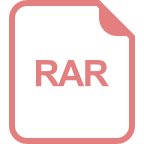







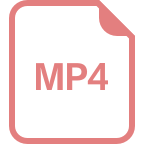