用jsp编写一个宠物增加修改删除代码
时间: 2023-08-02 07:11:43 浏览: 89
好的,以下是一个简单的宠物信息增删改查的JSP代码示例:
首先是添加宠物信息的代码,假设我们有一个Pet表,其中有id、name、age、type和gender等字段:
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="java.sql.*" %>
<%
request.setCharacterEncoding("UTF-8"); // 设置请求编码为UTF-8
String name = request.getParameter("name"); // 获取表单提交的数据
String age = request.getParameter("age");
String type = request.getParameter("type");
String gender = request.getParameter("gender");
Connection conn = null;
PreparedStatement stmt = null;
try {
Class.forName("com.mysql.jdbc.Driver"); // 加载MySQL驱动
// 建立数据库连接
String url = "jdbc:mysql://localhost:3306/test?useUnicode=true&characterEncoding=UTF-8&serverTimezone=UTC";
String user = "root";
String password = "123456";
conn = DriverManager.getConnection(url, user, password);
// 构造SQL语句
String sql = "INSERT INTO Pet(name, age, type, gender) VALUES(?, ?, ?, ?)";
stmt = conn.prepareStatement(sql);
// 设置参数
stmt.setString(1, name);
stmt.setInt(2, Integer.parseInt(age));
stmt.setString(3, type);
stmt.setString(4, gender);
// 执行SQL语句
int rows = stmt.executeUpdate();
if (rows > 0) {
out.print("添加成功!");
} else {
out.print("添加失败!");
}
} catch (Exception e) {
e.printStackTrace();
} finally {
// 关闭数据库连接
try {
if (stmt != null) {
stmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
%>
```
然后是修改宠物信息的代码,假设我们要根据id来修改宠物信息:
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="java.sql.*" %>
<%
request.setCharacterEncoding("UTF-8"); // 设置请求编码为UTF-8
int id = Integer.parseInt(request.getParameter("id")); // 获取表单提交的数据
String name = request.getParameter("name");
String age = request.getParameter("age");
String type = request.getParameter("type");
String gender = request.getParameter("gender");
Connection conn = null;
PreparedStatement stmt = null;
try {
Class.forName("com.mysql.jdbc.Driver"); // 加载MySQL驱动
// 建立数据库连接
String url = "jdbc:mysql://localhost:3306/test?useUnicode=true&characterEncoding=UTF-8&serverTimezone=UTC";
String user = "root";
String password = "123456";
conn = DriverManager.getConnection(url, user, password);
// 构造SQL语句
String sql = "UPDATE Pet SET name=?, age=?, type=?, gender=? WHERE id=?";
stmt = conn.prepareStatement(sql);
// 设置参数
stmt.setString(1, name);
stmt.setInt(2, Integer.parseInt(age));
stmt.setString(3, type);
stmt.setString(4, gender);
stmt.setInt(5, id);
// 执行SQL语句
int rows = stmt.executeUpdate();
if (rows > 0) {
out.print("修改成功!");
} else {
out.print("修改失败!");
}
} catch (Exception e) {
e.printStackTrace();
} finally {
// 关闭数据库连接
try {
if (stmt != null) {
stmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
%>
```
最后是删除宠物信息的代码,同样是根据id来删除:
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="java.sql.*" %>
<%
request.setCharacterEncoding("UTF-8"); // 设置请求编码为UTF-8
int id = Integer.parseInt(request.getParameter("id")); // 获取表单提交的数据
Connection conn = null;
PreparedStatement stmt = null;
try {
Class.forName("com.mysql.jdbc.Driver"); // 加载MySQL驱动
// 建立数据库连接
String url = "jdbc:mysql://localhost:3306/test?useUnicode=true&characterEncoding=UTF-8&serverTimezone=UTC";
String user = "root";
String password = "123456";
conn = DriverManager.getConnection(url, user, password);
// 构造SQL语句
String sql = "DELETE FROM Pet WHERE id=?";
stmt = conn.prepareStatement(sql);
// 设置参数
stmt.setInt(1, id);
// 执行SQL语句
int rows = stmt.executeUpdate();
if (rows > 0) {
out.print("删除成功!");
} else {
out.print("删除失败!");
}
} catch (Exception e) {
e.printStackTrace();
} finally {
// 关闭数据库连接
try {
if (stmt != null) {
stmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
%>
```
另外,还需要编写一个查询宠物信息的代码,用于显示宠物列表、编辑宠物信息等功能。具体实现方式可以使用JDBC连接数据库,构造SQL语句查询Pet表中的数据,并将结果以表格的形式显示在页面上。
阅读全文
相关推荐
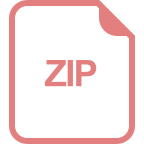
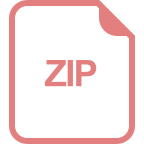
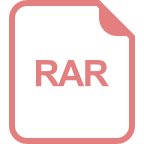
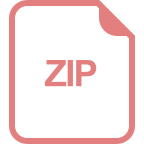
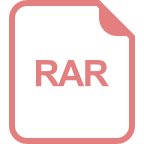
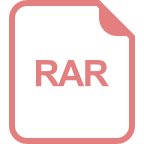
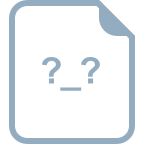
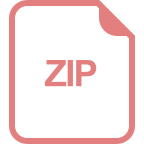
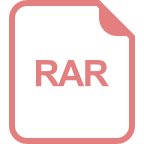
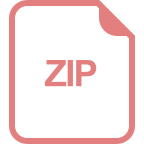
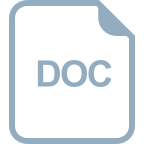
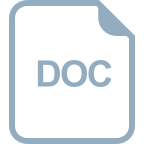
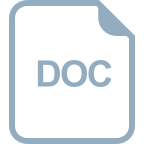
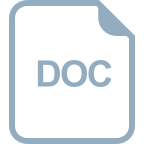
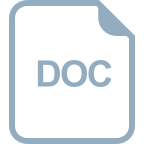
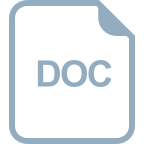
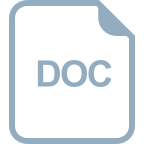